Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial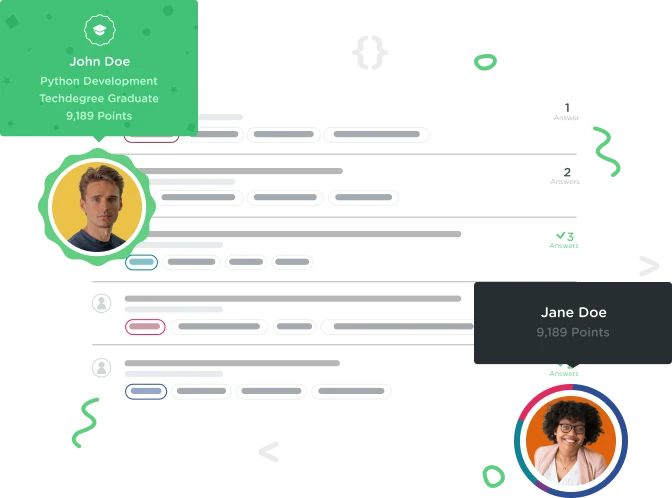

Syed Abbas
4,762 PointsObjects and variables
Hi I am new to object oriented programming. I have a confusion in my mind about objects. When we create an object of a certain type, we do it by using 'new' keyword and then use the object. On the other hand when we declare a variable of a class, we don't use 'new' keyword and still carry on using the variable. I hope I have made it clear what I am asking.
5 Answers
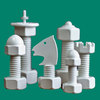
Steven Parker
230,274 PointsIt sounds like you already have a grasp of the concepts.
Variables are created ("declared") just by giving a type and their names. You can initialize a variable by extending the declaration with an assignment operator ("=
") and the value you want it to have.
If you want a variable to be a reference to a newly-created object, you use the "new" keyword and call the object's constructor.
int count; // declare a variable but don't give it a value
int size = 4; // declare and initialize the variable
count = 3; // assign a value to the variable previously declared
Widget thing; // declare a variable for an object but don't create one
Widget whatsit = new Widget(); // declare and initializes the variable, creating a new object
thing = new Widget(); // create a new object and assign the variable previously declared
ADDENDUM:
There's always exceptions, but typically you create an object to store something. For example, you might have a class named "Student" which has properties like name, id, major, courses, etc. You might instantiate (create) an object of this class so you could assign a specific name to the student, and/or any of the other properties.
As you proceed through your course the examples used in it should help make the concept more clear.

Syed Abbas
4,762 PointsThank you for your reply. When exactly is it that we need to create an object to initialise the variable? As I understand that we can use a variable without initialising it. For example, when an array is declared, the name of can be used to find the the length by using .Length next to the name. Do we create an object when we need it to perform something?
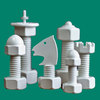
Steven Parker
230,274 PointsI added to my answer.

Syed Abbas
4,762 PointsThank you very much.
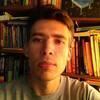
Daniel Tkach
7,608 PointsHi Syed. And Steven will help saying how accurate this is. This is how I understand it.
You use the keyword 'new' when you need to reserve a new space in memory, for a totally different object/variable. You don't use new when you are going to use that variable as a 'synonym'.
Objects contain memory address not the actual "data" so if you have:
Person student = new Person(); //new object in memory. Here you are doing three things at once: //1-declaring a variable, 2-creating a new object and 3- assigning it to the variable.
Person child; // empty variable, it can hold any object, but I'm not creating a new object. child = student; // now child points to the same data as student, they are synonyms, "child" and "student" are equivalent.
So here child and student point to the same object. I did not use 'new' for 'child' because I did not want to create a new object in memory, just a variable to point to an object I had already created, to use it as a synonym in a different section of my program.
If Steven or someone else approves this, I think it's a great way to understand it lol

Syed Abbas
4,762 PointsThank you for your answer.
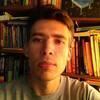
Daniel Tkach
7,608 PointsYou are welcome Syed. I'd appreciate a point if you are so kind. Thank you.