Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial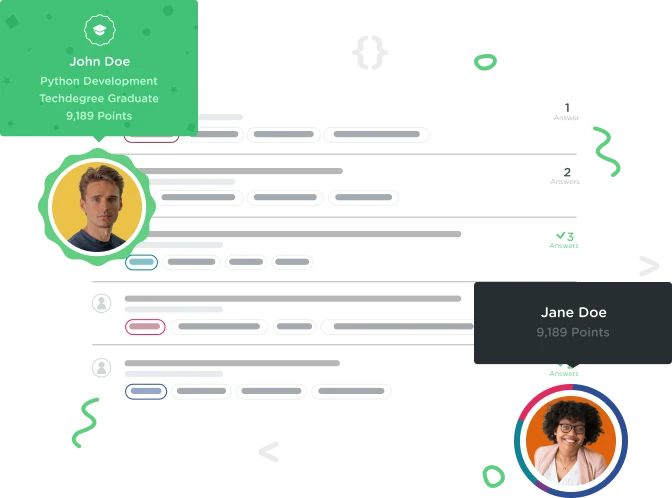

Uriah Nevins
1,121 PointsObjects, Classes and Parameters.
I am new to java, and I'm having a bit of confusion concerning objects and classes. I think I might just need it reworded a bit. What is a class? What is an object? Why and how do we use them?
1 Answer
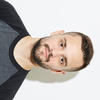
jacobproffer
24,604 PointsA class is an object factory. It defines the variables and methods understood by an instance of that class. When we declare a class, we are actually declaring two thing: The class itself and the objects that it is responsible for allocating at runtime. An "object" refers to a particular instance of the class.
If you're also confused on the difference between a parameter and an argument, let me break it down:
public class Helper {
public static String myString(String example) {
return example;
}
}
Above, the myString method takes a String as a parameter. A parameter is the name of a piece of information that is passed into a method. Once this method is called, it will return whatever String is passed in as an argument.
public class Example {
public static void main(String[] args) {
String test = Helper.myString("Jacob");
System.out.println(test);
}
}
To illustrate this, I've passed in the the argument "Jacob" into the myString method. It will store this value into a String named test.