Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial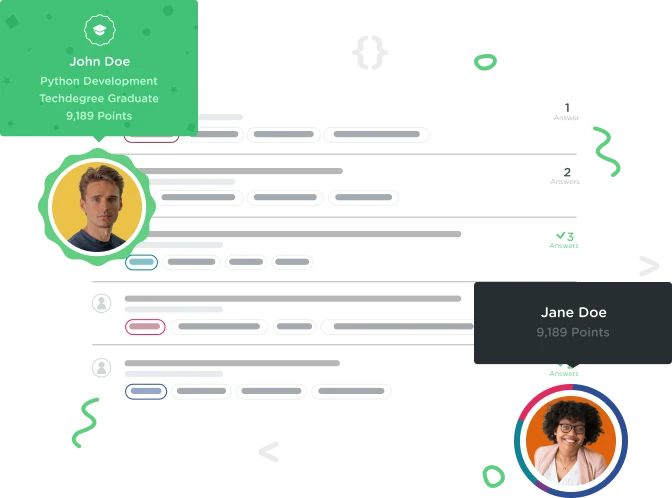

Raphael Varquez
4,758 Pointsobjects.swift answers plz
Don't know what's wrong with this code.
struct Location {
let latitude: Double
let longitude: Double
}
class Business {
let name: String
let location: Location
init(name: String, location: Location) {
self.name = name
self.location = Location(latitude: Double, longitude: Double)
}
}
let someBusiness: Business
1 Answer

Ghareisa Al-Kuwari
1,465 PointsIn init, you should not create an instance of Location as it is supposed to be already created, then passed as an argument to init.
To understand the difference, if we do want to create an instance of Location in init, it should be
init(name: String, latitude: Double, longitude: Double) {
self.name = name
self.location = Location(latitude: Double, longitude: Double)
}
Then when creating an instance of Business, you should give values to the arguments of init which are name and location, and here we do create an instance of Location, which is then directly passed an an init argument of type Location
let someBusiness = Business(name: "Business name", location: Location(latitude: 133.55, longitude: 23.2) )
The whole code should be
struct Location {
let latitude: Double
let longitude: Double
}
class Business {
let name: String
let location: Location
init(name: String, location: Location) {
self.name = name
self.location = location
}
}
let someBusiness = Business(name: "Business name", location: Location(latitude: 133.55, longitude: 23.2) )