Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial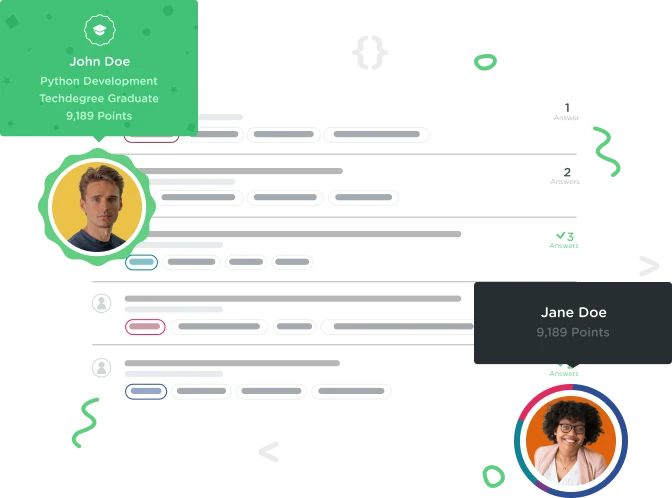

Andrew Ge
2,158 PointsObtaining Unknown Dictionary Elements
How do I get the first key of a dictionary, if I don't know what's in the dictionary?
Here's my code, where I assumed that "Kenneth Love" would be a key.
Even though I used this method, I think it should have worked...?
1 Answer
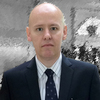
Nathan Tallack
22,160 PointsDictionaries are unordered by default (there is a ordered dictionary type but you are not learning that here).
So there is no "first" key as such.
What you can do is get a list of keys via the keys method. If your dictionary is named my_dict you could get a list of keys via my_dict.keys() which returns a list. You could iterate over that list.
Something like this.
my_dict = { "title1": "book1", "title2": "book2", "title3": "book3" }
for key in my_dict.keys():
print(my_dict[key])
That would result in this output.
book2
book1
book3
Remember, unordered. :)
Andrew Ge
2,158 PointsAndrew Ge
2,158 PointsThank you!
Chris Freeman
Treehouse Moderator 68,454 PointsChris Freeman
Treehouse Moderator 68,454 PointsAdditionally, when a
dict
is used in a iterator context like afor
loop, the default action is to iterate over thedict
keys. So the following are equivalent:To complete the topic, you can iterate over the values of a
dict
usingmy_dict.values()
, and you can iterate over a tuples of key / value pairs usingmy_dict.items()
.Nathan Tallack
22,160 PointsNathan Tallack
22,160 PointsOh wow. I did not know that the default action was to use the keys method. That is awesome.
I really should start reading the header files for some of these types so that I can learn cool things like that. But there is just so much content here on TT that I hardly find time to do any research myself. :P