Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial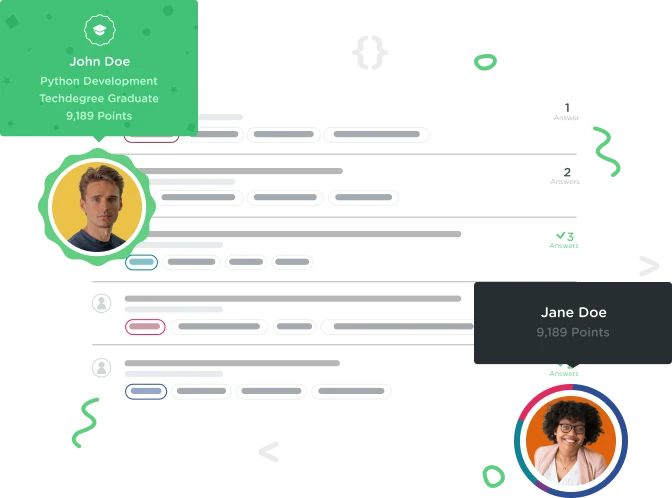

Daven Hietala
8,040 PointsOdd and even backgroundColor is not working.
I have been examining my code for about an hour now and still cannot see what I am doing wrong. The backgroundColor is supposed to turn lightgray and is not responding to my code.
Here is what I have so far..
const myList = document.getElementsByTagName('li');
for (let i = 0; i < myList.length; i += 1) {
myList[i].style.color = 'purple';
}
const errorNotPurple = document.querySelectorAll('.error-not-purple');
for (let i = 0; i < myList.length; i += 1) {
errorNotPurple[i].style.color = 'red';
}
const evens = document.querySelectorAll('li:nth-child(odd)');
for (let i = 0; i < evens.length; i += 1) {
evens[i].style.backgroundColor = 'lightgray';
}
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<h1 id="myHeading">JavaScript and the DOM</h1>
<p>Making a web page interactive</p>
<p title="label">Things that are purple:</p>
<ul>
<li>grapes</li>
<li class="error-not-purple">oranges</li>
<li>amethyst</li>
<li>lavender</li>
<li class="error-not-purple">fire trucks</li>
<li class="error-not-purple">snow</li>
<li>plums</li>
</ul>
<script src="app.js"></script>
</body>
</html>
@import 'https://fonts.googleapis.com/css?family=Lato:400,700';
body {
color: #484848;
font-family: 'Lato', sans-serif;
padding: .45em 2.65em 3em;
line-height: 1.5;
}
h1 {
margin-bottom: 0;
}
h1 + p {
font-size: 1.08em;
color: #637a91;
margin-top: .5em;
margin-bottom: 2.65em;
padding-bottom: 1.325em;
border-bottom: 1px dotted;
}
ul {
padding-left: 0;
list-style: none;
}
li {
padding: .45em .5em;
margin-bottom: .35em;
display: flex;
align-items: center;
}
input,
button {
font-size: .85em;
padding: .65em 1em;
border-radius: .3em;
outline: 0;
}
input {
border: 1px solid #dcdcdc;
margin-right: 1em;
}
div {
margin-top: 2.8em;
padding: 1.5em 0 .5em;
border-top: 1px dotted #637a91;
}
p.description,
p:nth-of-type(2) {
font-weight: bold;
}
/* Buttons */
button {
color: white;
background: #508abc;
border: solid 1px;
border-color: rgba(0, 0, 0, .1);
cursor: pointer;
}
button + button {
margin-left: .5em;
}
p + button {
background: #52bab3;
}
.list button + button {
background: #768da3;
}
.list li button + button {
background: #508abc;
}
li button:first-child {
margin-left: auto;
background: #52bab3;
}
.list li button:last-child {
background: #768da3;
}
li button {
font-size: .75em;
padding: .5em .65em;
}
I would really appreciate any help! I do not understand what I am doing wrong here. Thank you in advance.
3 Answers

KRIS NIKOLAISEN
54,971 PointsIf you check the console you'll find: Uncaught TypeError: Cannot read property 'style' of undefined for this line:
errorNotPurple[i].style.color = 'red';
This is because you are looping through i < myList.length when you only have three list items in errorNotPurple. If you change to i < errorNotPurple.length then the rest of your script will execute.

Daven Hietala
8,040 PointsThank you for your time Kris. What you have told me makes sense pertaining to this question. I am a bit confused as to why the code worked before on the previous video when it was like this.
const myList = document.getElementsByTagName('li');
for (let i = 0; i < myList.length; i += 1) {
myList[i].style.color = 'purple';
}
const errorNotPurple = document.querySelectorAll('.error-not-purple');
for (let i = 0; i < myList.length; i += 1) {
errorNotPurple[i].style.color = 'red';
}
The code still ran at that point. Once I added the evens variable to the code, I had the current issue. Why is it that the code worked before?
I hope this isn't an annoying question. My hope is to fully understand this lesson. Again, thank you Kris.
Zachary Danz
Front End Web Development Techdegree Graduate 15,024 PointsZachary Danz
Front End Web Development Techdegree Graduate 15,024 PointsJust wanted to say that you helped solve my problem as well! Thank you