Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial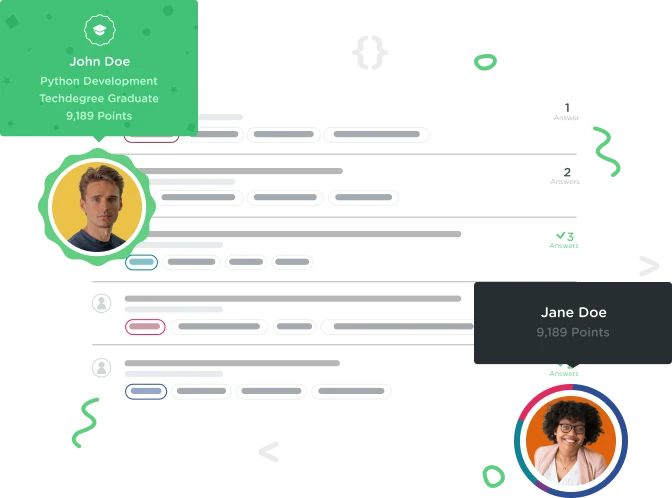

John Weland
42,478 PointsOdd error when clicking the daily button
I am getting an odd error when clicking the daily button
3701-3701/johnweland.me.stormy E/AndroidRuntime﹕ FATAL EXCEPTION: main
Process: johnweland.me.stormy, PID: 3701
java.lang.NullPointerException: Attempt to invoke virtual method 'void android.widget.ImageView.setImageResource(int)' on a null object reference
at johnweland.me.stormy.adapters.DayAdapter.getView(DayAdapter.java:60)
at android.widget.AbsListView.obtainView(AbsListView.java:2347)
at android.widget.ListView.makeAndAddView(ListView.java:1864)
at android.widget.ListView.fillDown(ListView.java:698)
at android.widget.ListView.fillFromTop(ListView.java:759)
at android.widget.ListView.layoutChildren(ListView.java:1673)
at android.widget.AbsListView.onLayout(AbsListView.java:2151)
at android.view.View.layout(View.java:15671)
at android.view.ViewGroup.layout(ViewGroup.java:5038)
at android.widget.RelativeLayout.onLayout(RelativeLayout.java:1076)
at android.view.View.layout(View.java:15671)
at android.view.ViewGroup.layout(ViewGroup.java:5038)
at android.widget.FrameLayout.layoutChildren(FrameLayout.java:579)
at android.widget.FrameLayout.onLayout(FrameLayout.java:514)
at android.view.View.layout(View.java:15671)
at android.view.ViewGroup.layout(ViewGroup.java:5038)
at android.widget.LinearLayout.setChildFrame(LinearLayout.java:1703)
at android.widget.LinearLayout.layoutVertical(LinearLayout.java:1557)
at android.widget.LinearLayout.onLayout(LinearLayout.java:1466)
at android.view.View.layout(View.java:15671)
at android.view.ViewGroup.layout(ViewGroup.java:5038)
at android.widget.FrameLayout.layoutChildren(FrameLayout.java:579)
at android.widget.FrameLayout.onLayout(FrameLayout.java:514)
at android.view.View.layout(View.java:15671)
at android.view.ViewGroup.layout(ViewGroup.java:5038)
at android.view.ViewRootImpl.performLayout(ViewRootImpl.java:2086)
at android.view.ViewRootImpl.performTraversals(ViewRootImpl.java:1843)
at android.view.ViewRootImpl.doTraversal(ViewRootImpl.java:1061)
at android.view.ViewRootImpl$TraversalRunnable.run(ViewRootImpl.java:5885)
at android.view.Choreographer$CallbackRecord.run(Choreographer.java:767)
at android.view.Choreographer.doCallbacks(Choreographer.java:580)
at android.view.Choreographer.doFrame(Choreographer.java:550)
at android.view.Choreographer$FrameDisplayEventReceiver.run(Choreographer.java:753)
at android.os.Handler.handleCallback(Handler.java:739)
at android.os.Handler.dispatchMessage(Handler.java:95)
at android.os.Looper.loop(Looper.java:135)
at android.app.ActivityThread.main(ActivityThread.java:5254)
at java.lang.reflect.Method.invoke(Native Method)
at java.lang.reflect.Method.invoke(Method.java:372)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:903)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:698)
the only file that's 'mine' (not built by AS) is the Day Adapter line:60
holder.iconImageView.setImageResource(day.getIconId());
3 Answers

Sam Wilskey
3,420 PointsIf I had to guess since I don't have access to all of the code. It would be that you did not set holder.iconImageView.
try this line inside of the if(convertView == null) statement
holder.iconImageView = (ImageView) convertView.findViewById(R.id.iconImageView);
The error is coming from a nullPointerException which means that the object you are trying to reference is null. Which would mean that you forgot initialize that object.
Edit:
I found the problem looking through the full code. The problem is that the id of what you are trying to access is not iconImageView but instead circleImageView. You are technically initializing the object holder.iconImageView but since the id does not match an id inside of the inflated daily_list_item it is just being initialized to null.
For future NullPointerExeptions my suggestion to debugging is to run the program inside of android studio's debugger and add a break point at the line number for the error. Since the error was with setting a imageResource on a Image view it is safe to assume that the ImageView is null in that case you need to just go through the code and see why it would be null. In this case just a missused ID.
Hope this helps.

Daniel Musser
3,711 PointsI'm having a very similar issue with getting a null pointer exception, and when I try to run the debugger with the breakpoint at the line number that contains the error, it does show that the holder.iconImageView is null, but I can't figure out for the life of me, why it would be, since I basically just copied the code that Ben shows in the videos exactly.
The top portion of the error I'm getting is:
FATAL EXCEPTION: main
Process: com.example.danielmusser.stormy, PID: 11436
java.lang.NullPointerException: Attempt to invoke virtual method 'void android.widget.ImageView.setImageResource(int)' on a null object reference
at com.example.danielmusser.stormy.adapters.DayAdapter$override.getView(DayAdapter.java:61)
at com.example.danielmusser.stormy.adapters.DayAdapter$override.access$dispatch(DayAdapter.java)
at com.example.danielmusser.stormy.adapters.DayAdapter.getView(DayAdapter.java:0)
And this is what my dayAdapter class looks like:
package com.example.danielmusser.stormy.adapters;
import android.content.Context;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.ImageView;
import android.widget.TextView;
import com.example.danielmusser.stormy.R;
import com.example.danielmusser.stormy.weather.Day;
public class DayAdapter extends BaseAdapter {
private Context mContext;
private Day[] mDays;
public DayAdapter(Context context, Day[] days) {
mContext = context;
mDays = days;
}
@Override
public int getCount() {
return mDays.length;
}
@Override
public Object getItem(int position) {
return mDays[position];
}
@Override
public long getItemId(int position) {
return 0; // won't be used, but you can tag items for easy reference
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder holder;
if (convertView == null) {
// brand new
convertView = LayoutInflater.from(mContext).inflate(R.layout.daily_list_item, null);
holder = new ViewHolder();
holder.iconImageView = (ImageView) convertView.findViewById(R.id.iconImageView);
holder.temperatureLabel = (TextView) convertView.findViewById(R.id.temperatureLabel);
holder.dayLabel = (TextView) convertView.findViewById(R.id.dayNameLabel);
convertView.setTag(holder);
} else {
holder = (ViewHolder) convertView.getTag();
}
Day day = mDays[position];
holder.iconImageView.setImageResource(day.getIconId());
holder.temperatureLabel.setText(day.getTemperatureMax() + "");
holder.dayLabel.setText(day.getDayOfTheWeek());
return convertView;
}
private static class ViewHolder {
ImageView iconImageView; // public by default
TextView temperatureLabel;
TextView dayLabel;
}
}
Does anybody have any idea on what I'd need to do to fix it?

Daniel Musser
3,711 PointsSOLVED IT!
I used the debugger to check that all of the variables were getting populated with info and they were, so I eventually saw that the holder.iconImageView
was null even after the line where it should have been populated with a resource id. So I checked the id of the iconImageView
in the daily_list_item.xml
and iconImageView
had a capital "i" at the beginning. So I fixed that, and then my code worked!
John Weland
42,478 PointsJohn Weland
42,478 PointsHere is that entire DayAdapter class
here is a link to my repo of it. https://github.com/johnweland/stormy/tree/ListViews%26RecyclerViews
Sam Wilskey
3,420 PointsSam Wilskey
3,420 PointsEdited after looking through your code. I kept my original response because that can be the reason for the error in many cases in this situation.
John Weland
42,478 PointsJohn Weland
42,478 PointsThanks mate!
Ken Wagner
7,298 PointsKen Wagner
7,298 PointsThank you Sam!!!!
I've been trying to figure it out for the last couple of days. Cross-referencing the downloadable files didn't help, because they contain the same line of code.
Thanks again!