Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial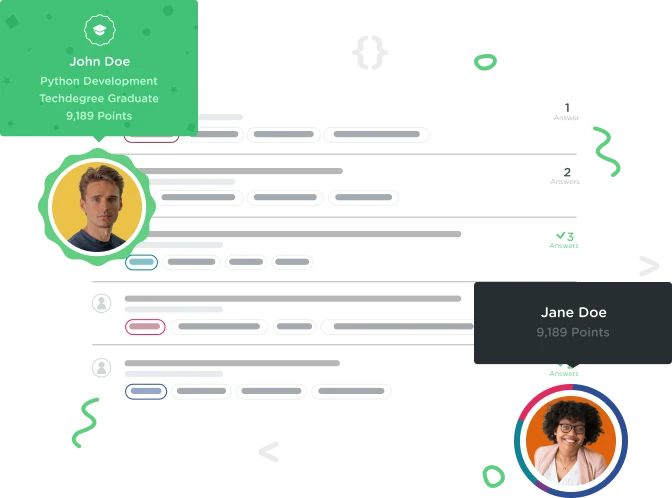
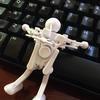
Michelle Wood
1,667 Pointsodd_even.py
code passes challenge. when i run the code in workspaces, no errors - and no output. what am i missing to run and see output?
thanks much.
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
start = 5
while start == True:
random_number = random.randint(1, 99)
number = even_odd(random_number)
if number == True:
print(" {} is even".format(random_number))
else:
print(" {} is odd".format(random_number))
start -=1
3 Answers
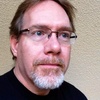
Chris Freeman
Treehouse Moderator 68,423 PointsThe while
loop will never run because
start == True
is never True
. While start can be "truthy" it wii never be equal to
True. Simply use the "truthiness" is
start`:
while start:
This extends to using if number:
instead as well.
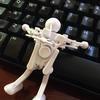
Michelle Wood
1,667 PointsThanks Chris! Another logic question... I understand that if num % 2 is 0, then we've got an even num. Trying to understand the use of 'not'. Is the below saying 'return not 0' -- meaning return true? And if so, then how does even_odd function ever indicate num is an even number, which would be returning a 0... ?
return not num % 2
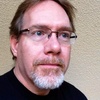
Chris Freeman
Treehouse Moderator 68,423 PointsIt has to do with context.
# True and False can be cast as integers
>>> int(True)
1
>>> int(False)
0
# and can be compared with integers
>>> 1 == True
True
>>> 0 == False
True
# but are Not integers
>>> 0 is False
False
>>>
# they are Boolean
>>> type(False)
<class 'bool'>
# modulo % returns an int
>>> 5 % 2
1
# the 'not' says "return the opposite of the truthiness of the expression"
>>> not 5 % 2
False
>>> not 1
False
So because the not
casts its output as Boolean, even_odd
returns a Boolean.
Hope that makes sense.
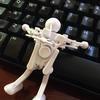
Michelle Wood
1,667 PointsThank you - very helpful!