Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial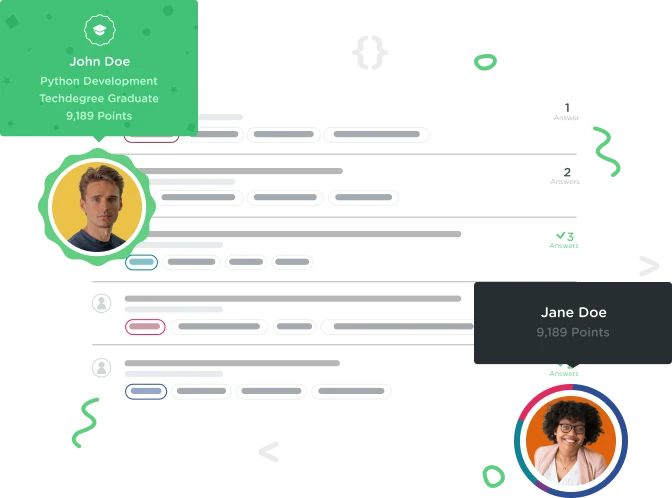

chelseacheevers
13,786 PointsOff Platform Challenge Help Request (Flexbox, primarily)
Hello everyone,
I have been studying with Treehouse for sometime, in order to become a dev. My partner is also a dev and is helping me by giving me code challenges, but in a dev way, which is to say they believe I should be figuring things out on my own. They will either give me the answer, or not, but there's no "teaching" or explanation, and unfortunately, even though I do understand a lot of it, none of this comes naturally to me, and I need more help when they did for learning. Luckily, I remembered that I am still paying for this membership which includes a community of support, so I am hoping to get some here.
So, now that the lengthy background story has been shared, here's the code issue I am having. First, I will introduce the entire challenge for context:
"From main branch, please create a new branch and name it "chels-dev" -Please reproduce the attached example in assets folder using the template below -You are required to create 2 different examples separately: 1 with using only GRID and the one with only Flexbox. -Once the 2 tasks are complete, please use javascript to create an animation: On mouseover, please first scale the cell by 25% THEN translate the cell to the right by 50px. ---The animations should be smooth. -Once the task is complete, please push your work to the dev branch"
Ok, so that's the whole challenge. Where I am struggling specifically is with Flexbox. Grid was pretty straightforward to do, and I have a fair understanding of DOM manipulation, which I am reviewing and working on now, but what I am hoping to get from this post is help with Flexbox. I will include the code below, and then outline my current struggle:
HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="./index.css">
<title>Project one</title>
</head>
<body>
<img src="assets/example.jpeg" alt="template example">
<h3>With GRID</h3>
<section class="wrapper-1">
<div class="shared header head-g">header</div>
<div class="shared menu menu-g">menu</div>
<div class="shared hero hero-g">hero</div>
<div class="shared main main-g">main</div>
<div class="shared banner bann-g">banner</div>
<div class="shared extra ext-g">extra</div>
<div class="shared image image-g">image</div>
</section>
<br>
<section>
hello
</section>
</section>
<h3>With Flexbox</h3>
<section class="wrapper-2">
<section class="row-1">
<div class="shared header head-f">header</div>
<div class="shared menu menu-f">menu</div>
<div class="shared hero hero-f">hero</div>
</section>
<section class="wrapper-3">
<section class="row-2">
<div class="shared menu hidden-menu">menu</div>
</section>
<section class="row-3">
<div class="shared main main-f">main</div>
<div class="shared banner bann-f">banner</div>
<section class="wrapper-4">
<div class="shared extra extra-f">extra</div>
<div class="shared image img-f">image</div>
</section>
</section>
</section>
</section>
<script src="./index.js"></script>
</body>
</html>
CSS:
/* Base Styles */
body {
margin: 0;
font-family: "Avenir", sans-serif;
}
.shared {
display: flex;
color: white;
padding: 20px;
font-size: 20px;
text-transform: uppercase;
justify-content: center;
align-items: center;
}
img {
width: 900px;
height: 500px;
}
p {
margin: 0;
}
.header {
background: #0f1629;
}
.menu {
background: #ed3d4d;
}
.hero {
background: #4c86c6;
}
.main {
background: #24334b;
}
.banner {
background: #f9d169;
}
.extra {
background: #51bd97;
}
.image {
background: #c1e5e5;
}
/* Grid */
.head-g { grid-area: head; }
.menu-g { grid-area: menu; }
.hero-g { grid-area: hero; }
.main-g { grid-area: main; }
.bann-g { grid-area: ban; }
.ext-g { grid-area: extra; }
.image-g { grid-area: img; }
.wrapper-1 {
display: grid;
grid-template-columns: repeat(4, 1fr);
grid-auto-rows: minmax(75px, auto);
grid-template-areas:
"head head head head"
"menu menu menu menu"
"hero hero hero hero"
"hero hero hero hero"
"hero hero hero hero"
"main main main main"
"main main main main"
"main main main main"
"ban ban ban ban"
"ban ban ban ban"
"extra extra extra extra"
"extra extra extra extra"
"img img img img"
"img img img img";
}
@media screen and (min-width: 700px) {
.wrapper-1 {
display: grid;
grid-template-columns: repeat(4, 1fr);
grid-auto-rows: minmax(75px, auto);
grid-template-areas:
"head head head head"
"hero hero hero hero"
"hero hero hero hero"
"hero hero hero hero"
"hero hero hero hero"
"menu main main main"
"menu main main main"
"menu main main main"
"menu main main main"
"menu ban ban ban"
"menu ban ban ban"
"menu extra extra img"
"menu extra extra img";
}
}
@media screen and (min-width: 960px) {
.wrapper-1 {
display: grid;
grid-template-columns: repeat(4, 1fr);
grid-auto-rows: minmax(75px, auto);
grid-template-areas:
"head menu menu menu"
"hero hero hero hero"
"hero hero hero hero"
"hero hero hero hero"
"hero hero hero hero"
"hero hero hero hero"
"main main . img"
"main main . extra"
"ban ban ban ban";
}
}
/* Flexbox */
/* Mobile */
.hidden-menu {
display: none;
}
.row-1 {
display: flex;
flex-flow: wrap;
width: 100%;
}
.head-f {
width: 100%;
height: 45px;
}
.menu-f {
width: 100%;
height: 45px;
}
.hero-f {
width: 100%;
height: 175px
}
.row-3 {
display: flex;
flex-flow: wrap;
}
.row-3 .main-f {
width: 100%;
height: 175px;
}
.row-3 .bann-f {
width: 100%;
height: 90px;
}
.wrapper-4 {
width: 100%;
}
.wrapper-4 .extra-f {
width: 100%;
height: 90px;
}
.wrapper-4 .img-f {
width: 100%;
height: 90px;
}
/* Tablets */
@media screen and (min-width: 700px) {
.menu-f {
display: none;
}
.row-1 .hero-f {
height: 250px;
}
.wrapper-3 {
display: flex;
flex-flow: wrap;
flex: 1 1 0;
}
.hidden-menu {
display: flex;
}
.row-2 {
width: 25%;
/* height: 100%; */
}
.row-3 {
width: 75%;
}
.row-2 {
display: flex;
}
.row-2 .hidden-menu {
width: 100%;
}
.wrapper-4 {
display: flex;
}
.extra-f {
width: 33.33% !important;
}
.img-f {
width: 66.66% !important;
}
}
/* Desktop */
@media screen and (min-width: 960px) {
.row-2 {
display: none;
}
.row-1 {
display: flex;
flex-flow: wrap;
width: 100%;
}
.head-f {
width: 25% !important;
}
.menu-f {
width: 75% !important;
visibility: visible;
}
}
Ok, so I've included everything in case you want to run the files and take a look. I'm not sure if the asset image will load, but I included the Grid component, which is functional in all screen sizes if you would like to compare.
As you can see, I am struggling with the desktop media query. I think my trouble lies in having created a hidden menu and then trying to bring back the original menu in the desktop after hiding it in tablet. There are a few other quirky bugs that I am struggling to work out with this section as well, and I am anticipating meeting some challenges in "row 3" and "wrapper 4", but one mountain at a time... I would of course be very grateful of any solutions that can be provided, but most appreciative of anyone who would be able to explain what on my end is wrong, and why, and why the solution is correct. I know the second is a lot to ask on a semi-public forum, so it's not expected, honestly any guidance would be appreciated.
I am going to get going on the Javascript component and hopefully when I am done I will have some more answers on the Flexbox topic.
Thanks in advance, have a great day, and happy coding!
1 Answer
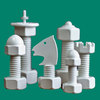
Steven Parker
231,248 PointsWhile not specific to your task, two free and fun learning resources that I found very useful when learning flexbox (and two more I discovered recently):
chelseacheevers
13,786 Pointschelseacheevers
13,786 PointsThank you very much!