Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial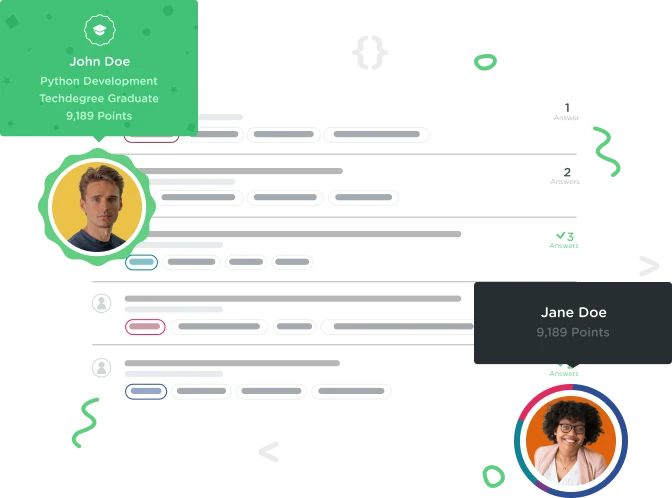

Alonzo Delk
6,991 PointsOk, I need some help on this one. As far the arrow function where is my mistake?
const greet => ('cool coders');
function greet(val) {
return `Hi, ${val}!`;
};
console.log(greet);
3 Answers
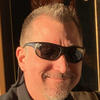
Peter Vann
36,427 PointsHi Alonzo!
A couple of issues:
1) You don't need the console.log statement (not part of the challenge).
2) You need to declare the function first, then call it (meaning you have to rearrange the code).
3) You have to declare the function as an arrow function.
This passes"
const greet = (val) => `Hi, ${val}!`; //Simple, shorthand arrow function syntax
greet('cool coders');
You could also code it this way:
const greet = (val) => {
return `Hi, ${val}!`;
}
greet('cool coders');
If you use the brackets, you need the return.
Keep in mind that the second code example is necessary if the code block has multiple lines of code, otherwise, you can shorten it to the first example.
I hope that helps.
Stay safe and happy coding!

Alonzo Delk
6,991 Pointsfunction greeting( name ) { const message = "Hello " + name; ; }
What am I missing in this problem, to return tp value stored
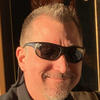
Peter Vann
36,427 PointsHi Alonzo!
First, this isn't an arrow function.
To properly use this type of function though, you'd need to use this:
function greeting( name ) {
const message = "Hello " + name;
return message;
}
You could also shortcut it to this (you don't have to use the variable):
function greeting( name ) {
return "Hello " + name;
}
An arrow function version would look like this, though:
const greeting = name => "Hello " + name;
Or
const greeting = (name) => {
return "Hello " + name;
}
And you would use/call it like this (either way):
const myGreeting = greeting("Alonzo");
console.log(myGreeting);
Which, of course, would log this to the console:
Hello Alonzo
I hope that helps.
Stay safe and happy coding!