Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial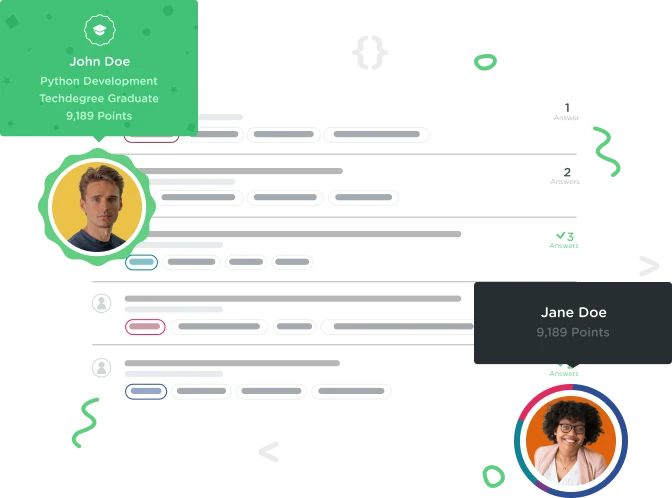

mamadou diene
Python Web Development Techdegree Student 1,971 PointsOK, I need you to finish writing a function for me. The function disemvowel takes a single word as a parameter and then
Help please. i need pointers on this one
char word = "treehouse"
vowels = ("a", "e", "i", "o", "u")
def disemvowel(word):
try:
word.remove(vowels).lower() or word.remove(vowels).upper()
except ValueError:
pass
return word
1 Answer
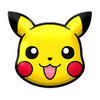
Valeriya Romashchenko
11,841 PointsHello!
Your code has several problems like:
- When you define the variable it should be one word : "char word" ===> "char_word";
- Be careful about indentation in python. At least it should look like:
char_word = "treehouse"
vowels = ("a", "e", "i", "o", "u")
def disemvowel(word):
try:
word.remove(vowels).lower() or word.remove(vowels).upper()
except ValueError:
pass
return word
- You store your vowel in the tuple, not in the list. I don't know did you made on purpose, or you intended to make a list:
python vowels = ["a", "e", "i", "o","u"]
-
Also, .remove() is a list method, and you apply it for the string. from python docs:
list.remove(x) Remove the first item from the list whose value is x. It is an error if there is no such item.
I don't see the reason to use here try-except block. You don't need to validate the users' input, check if it is string or integer etc. Here you can just iterate through the word and check if the letter is vowel or not. If it is a vowel - ignore, if not - keep the letter.
This challenge says that we can solve this task however we want. My code like this passed the challenge:
def disemvowel(word):
result_word = [] # I am creating an empty list where I am going to store letters that are not vowels
vowels = ["a", "e", "i", "o","u"] # list of vowels which is going to help find all vowels in the word
for letter in word: # I want to check every letter in the word and iterate with for loop through the word
if letter.lower() not in vowels: # I check if the letter not in the list of the vowels
result_word.append(letter) # if it is NOT, I append to my empty list the letter that is not in the vowels list
return ''.join(result_word) # I return the result list as a string by joining all elements in the list without any condition
Don't be discouraged! I am a different person and our logic can vary in the approaching the problem. If you can solve this with try-except block, share your code, please)