Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial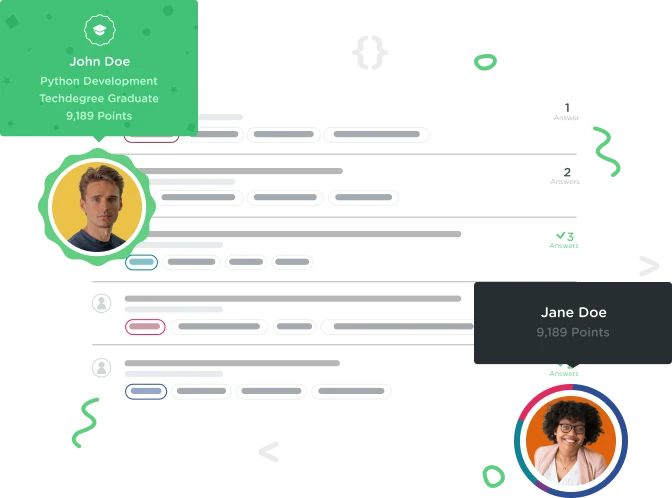
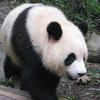
Eitay Zilbershmidet
3,942 PointsOkay, so let's use our new isFullyCharged helper method to change our implementation details of the charge method. Let's
Hello,
I can't figure this out, what's wrong?
public class GoKart { public static final int MAX_ENERGY_BARS = 8; private String mColor; private int mBarsCount;
public GoKart(String color) { mColor = color; mBarsCount = 0; }
public String getColor() { return mColor; }
public void charge() { mBarsCount = MAX_ENERGY_BARS; }
public boolean isBatteryEmpty() { return mBarsCount == 0; }
public boolean isFullyCharged() { return mBarsCount == MAX_ENERGY_BARS; }
}
public class GoKart {
public static final int MAX_ENERGY_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void charge() {
mBarsCount = MAX_ENERGY_BARS;
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_ENERGY_BARS;
while (!isFullyCharged()) {
return mBarsCount++;
}
}
}
7 Answers
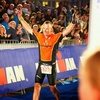
Steve Hunter
57,712 PointsHi Eitay,
OK, so in this challenge we want to amend the charge
method. At the moment, it looks like this:
public void charge() {
mBarsCount = MAX_ENERGY_BARS;
}
so it just sets the mBarsCount
member variable to be the value held in the constant, MAX_ENERGY_BARS
.
What the question wants is to use the helper method isFullyCharged()
to control a loop within the charge
method. Inside this loop, mBarsCount
will be incremented each time the code loops. The loop will stop depending on what comes back from isFullyCharged()
. Let's have a look at the method - we aren't going to change this code; it works just fine but we need to see what it does:
public boolean isFullyCharged() {
return mBarsCount == MAX_ENERGY_BARS;
}
This method compares the current value of mBarsCount
with the value in MAX_ENERGY_BARS
and will return a boolean
depending on whether the two values are equal, or not. So, if the GoKart is fully charged, the two values are equal, and the method will return true
. We want to the loop to stop when this happens.
So let's set up a while
loop. Just a basic one first; just a skeleton:
while(//some condition){
// change something
}
What do we want to change? The level of mBarsCount
- when do we want to stop doing this? When isFullyCharged()
returns true
. Let's incorporate that:
while(isFullyCharged()){
mBarsCount++;
}
Does that work for us? Not quite. Let's say that the GoKart is not fully charged. We want it to charge, right? But the while loop won't run because isFullyCharged()
will return false
. We want the condition in the loop to evaluate to true
so that the loop will run, then, when the GoKart is fully charged, the condition must change to false
so the loop stops. That's the exact opposite of what we have here. We can negate the condition using the NOT
operator, the exclamation mark, !
. That negates the condition so it will work as we want it to:
while(!isFullyCharged()){
mBarsCount++;
}
So, we need to replace the current charge
method's content with this loop:
public void charge() {
while(!isFullyCharged()){
mBarsCount++;
}
}
With this, the GoKart will charge one bar at a time until the GoKart reaches the capacity defined in MAX_ENERGY_BARS
.
Does that make sense? Let me know how you get on.
Steve.
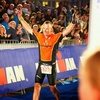
Steve Hunter
57,712 PointsHi there,
Have a read through this post and let me know if that helps you understand the concepts here.
If not, shout back and I'll go through it.
Steve.
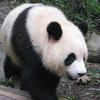
Eitay Zilbershmidet
3,942 PointsHi Steave,
Thank you for reply :) I have tried it but still, it doesn't work for me..
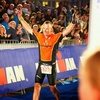
Steve Hunter
57,712 PointsOK - have you reset your code back to the start? Do that, and I'll type up an explanation now. Give me a minute!
Steve.

Pavlo Kochubei
15,009 PointsJust a test comment
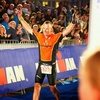
Steve Hunter
57,712 PointsThat's an answer, not a comment.
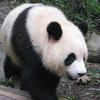
Eitay Zilbershmidet
3,942 PointsHi Steve,
Thank you so mach :) You explained very well. Now I understand that my mistake was very foolish. I located my loop above the isFullyCharged() so it didn't copiled.
Thank you again
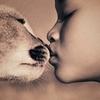
melissakeith
3,766 PointsI've reset my code and changed it to match the way you've done it here, but still get the same error?? Need help.
./GoKart.java:29: error: incompatible types: int cannot be converted to boolean return mBarsCount++; ^
public class GoKart {
public static final int MAX_ENERGY_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void charge() {
while (!isFullyCharged()){
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_ENERGY_BARS;
while (!isFullyCharged()){
return mBarsCount++;
}
}
}
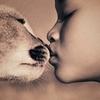
melissakeith
3,766 Pointsunfortunately this didn't keep the format so its difficult to see what I may be doing wrong
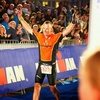
Steve Hunter
57,712 PointsHi Melissa,
You have a while loop inside the isFullyCharged
method. Remove that. This method just returns the comparison between mBarsCount
and MAX_BARS. The rest looks OK.
Steve.
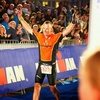
Steve Hunter
57,712 PointsSo, isFullyCharged
just returns the comparison:
public boolean isFullyCharged() {
return mBarsCount == MAX_ENERGY_BARS;
}
That was causing the error. That method returns a boolean
and you had it returning an int by using return mBarsCount++
.
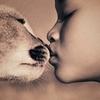
melissakeith
3,766 PointsThank you I apparently just changed too many things.