Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial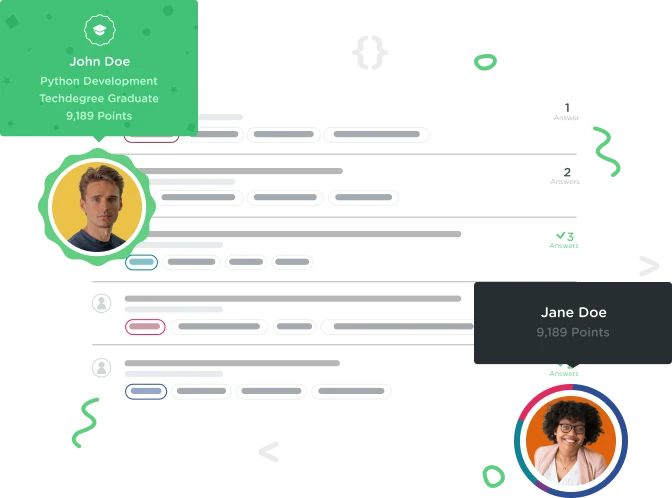

M. Brown
29,923 PointsOkay so this is the best I could do for task two after trying for a few days
I thought I would of naturally had an answer by now.
import java.util.List;
import java.util.ArrayList;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.Criteria;
public class LanguageRepository {
// Assume sessionFactory has been initialized properly by its default constructor
@SuppressWarnings("unchecked")
public SessionFactory {
private static final SessionFactory sessionFactory = new SessionFactory();
public List<Language> findAll() {
// Open session
Session session = sessionFactory.openSession();
// TODO: Create Criteria
Criteria criteria = session.createCriteria(Language.class);
// TODO: Get a list of all persisted Language entities
List<Language> languages = criteria.list();
// Close the session
session.close();
// Return the list
return languages;
}
}
}
1 Answer

Samuel Ferree
31,722 PointsYour code is mostly correct. You don't need to wrap the code in an inner class (which you've called SessionFactory) So I removed that, and then moved the suppress warnings annotation down to the findAll() method. If you wanted to suppress warnings for the entire class, you could have also put the annotation above the line 'public class LanguageRepository'
import java.util.List;
import java.util.ArrayList;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.Criteria;
public class LanguageRepository {
private static final SessionFactory sessionFactory = new SessionFactory();
@SuppressWarnings("unchecked")
public List<Language> findAll() {
// Open session
Session session = sessionFactory.openSession();
// TODO: Create Criteria
Criteria criteria = session.createCriteria(Language.class);
// TODO: Get a list of all persisted Language entities
List<Language> languages = criteria.list();
// Close the session
session.close();
// Return the list
return languages;
}
}