Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial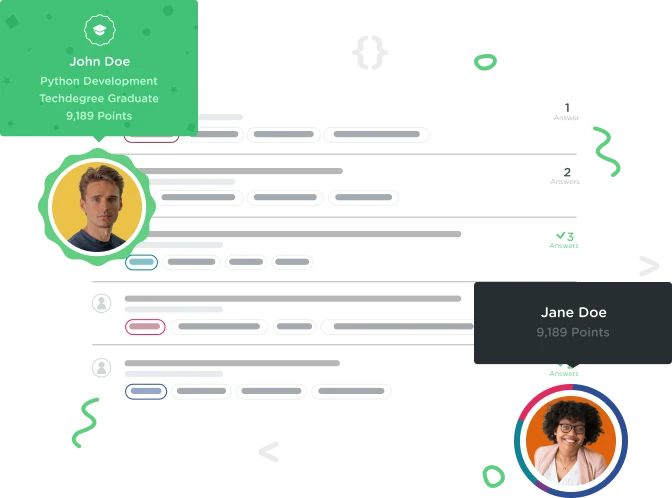

Bami Learn
914 PointsOn a new line use the on method to listen for the error event. Pass in a callback function with one parameter of error.
I can't seems to find what I'm doing wrong in this code.
var http = require("http");
http.get("http://teamtreehouse.com/chalkers.json", function(response){
console.log(response.statusCode);
});
var request = http.get;
request.on('error', function(error){});
6 Answers
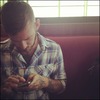
Erik McClintock
45,783 PointsBami,
You've got your variable request
assigned incorrectly. The first task shouldn't have let it pass how you wrote it, which is why there is confusion now. They want you to create a variable request
and assign it the result of your get request, which is retrieved from the filled out get request that was already present in the code. If you assign your request
variable in the correct spot, you will pass (i.e. your call to the on
method is correct).
You have:
var http = require("http");
http.get("http://teamtreehouse.com/chalkers.json", function(response){
console.log(response.statusCode);
});
var request = http.get;
request.on('error', function(error){});
You want:
var http = require("http");
// this is where you create/assign the 'request' variable the result of your get request
var request = http.get("http://teamtreehouse.com/chalkers.json", function(response){
console.log(response.statusCode);
});
request.on('error', function(error){});
Erik
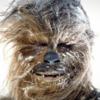
codingchewie
8,764 PointsSaw this when I got stuck but also noticed the age of this. The provided top voted answer uses var
but with ES6 you can code the request
with a const
:
const request = https.get("https://teamtreehouse.com/chalkers.json", response => {
console.log(response.statusCode);
});
Still new in learning but I believe instead of using:
request.on('error', function(error){});
you could use an arrow function:
request.on('error', error => {});
hope this helps the next person.
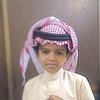
Ayman Omer
9,472 Points const https = require("https");
const request=https.get("https://teamtreehouse.com/chalkers.json", response => {
console.log(response.statusCode);
});
request.on('error',error=>{ })
```

Bami Learn
914 Pointsoh! Thanks alot Erik

vikram 11023
12,043 PointsOn a new line use the on method to listen for the error event. Pass in a callback function with one parameter of error.
const https = require("https");
var request = https.get("https://teamtreehouse.com/chalkers.json", response => { console.log(response.statusCode); });
request.on ('error',error => console.error('problem with request : $ {error.message}')); // callback functon with one parameter of error.
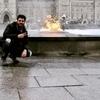
VENKATESH NALLA
15,919 Pointsconst https = require("https");
const request=https.get("https://teamtreehouse.com/chalkers.json", response => { console.log(response.statusCode);
}); request.on('error',error=>{ })