Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial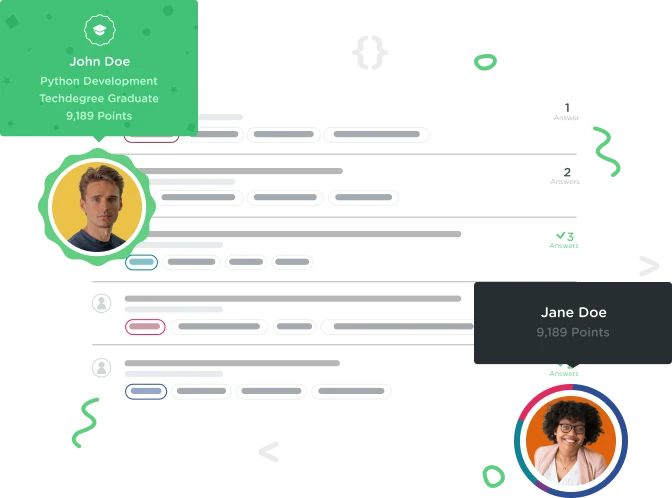

wylecordero
1,788 PointsOn dungeon_game.py I keep getting : TypeError: can only join an iterable
on pycharm here is the error:
Traceback (most recent call last): File "C:/Users/x3d3/Desktop/Wyle C Folders/PYTHON/dungeon_game.py", line 92, in <module> print("you can move {}".format(", ".join(get_moves))) # fill with available moves. TypeError: can only join an iterable

wylecordero
1,788 Pointshere is all of the code so far.
"""
======================================================================================================================
Notes: random.sample function , to make sure that our random selections selection does not
overlap. by doing that instead of: monster = None
door = None
player = None
return monster, door, player
you can: return random.sample(CELLS, 3), cells variable is the iterable.
Note: too many values to unpack error means you have extra elements that needs to be packed , use return
to return the extra variables.
======================================================================================================================
Program Needs:
# draw grid
# pick a random location for the player.
# pick random location for exit door.
# pick random location for the monster.
# draw player in the grid.
# take input for movement.
# move player, unless invalid move (past edges of grid)
# check for win/loss.
# clear scree and redraw grid.
======================================================================================================================
"""
import os
import random
# to create grid, grid is 5*5.
CELLS = [(0, 0), (1, 0), (2, 0), (3, 0), (4, 0),
(0, 1), (1, 1), (2, 1), (3, 1), (4, 1),
(0, 2), (1, 2), (2, 2), (3, 2), (4, 2),
(0, 3), (1, 3), (2, 3), (3, 3), (4, 3),
(0, 4), (1, 4), (2, 4), (3, 4), (4, 4)]
def clear_screen():
os.system('cls' if os.name == 'nt' else 'clear')
def get_locations():
return random.sample(CELLS, 3)
def move_player(player, move):
x, y = player
if move == "LEFT": # if move == LEFT, x-1.
X -= 1
if move == "RIGHT": # if move == RIGHT, x+1.
x += 1
if move == "UP": # if move == UP, y-1.
y -= 1
if move == "DOWN": # if move == DOWN, y+1.
y += 1
return x, y
def get_moves(player):
moves = ["LEFT", "RIGHT", "UP", "DOWN"] # get player's location.
x, y = player
if x == 0: # if player's x == 0, they cannot move left.
moves.remove("LEFT")
if x == 4: # if player's x == 4, they cannot move right.
moves.remove("RIGHT")
if y == 0: # if player's y ==0, they cannot move up.
moves.remove("UP")
if y == 4: # if player's y == 4, they cannot move down.
moves.remove("DOWN")
return x, y
return moves
# put outside of the while loop, because you do not want to relocate each time.
monster, door, player = get_locations()
while True:
valid_moves = get_moves(player)
clear_screen()
print("Welcome to the dungeon!")
print("You are currently in room {}".format(player)) # fill with player position
print("you can move {}".format(", ".join(valid_moves))) # fill with available moves.
print("Enter QUIT to quit")
move = input("> ")
move = move.upper()
if move == 'QUIT':
break
if move in valid_moves:
player = move_player(player, move)
else:
print("\n**Walls are hard!, do not run into them! **\n")
continue
# good move?, change the player position.
# bad move?, do not change anything.
# on the door? they win!
# on the monster? they lose!
# otherwise, loop back around.
1 Answer

Christian Mangeng
15,970 PointsHi Wyle,
the error is in the get_moves() function: besides returning moves (which is correct), you also return the player coordinates x and y.

wylecordero
1,788 PointsHello Christian,
You are correct , i do not know why i had the player coordinates , but it worked once that line was deleted. You are awsesome, thank you.
wylecordero
1,788 Pointswylecordero
1,788 Points