Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial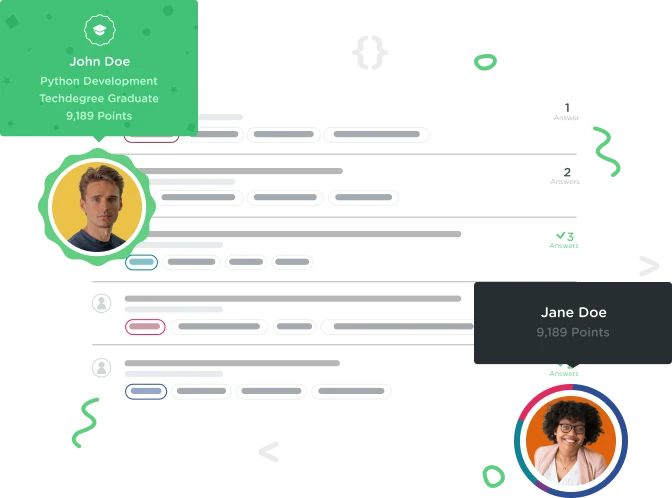

Edwin Walela
1,373 PointsOn submitting the form, the URL changes to "http://localhost:3000/?" and I remain on the Home page. Any help?
My handleSubmit Code snippet
handlesubmit = (e) => {
e.preventDefault();
let teacherName = this.name.value;
let teacherTopic = this.topic.value;
let path = `teachers/${teacherTopic}/${teacherName}`;
this.props.history.push(path)
}
3 Answers
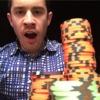
Reid Young
Courses Plus Student 14,209 PointsHave you tried pushing your path as /teachers/${teacherTopic}/${teacherName}
; with a forward slash in this template literal? Also, don't forget to reset the currentTarget!
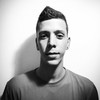
Jesus Mendoza
23,289 PointsCan we have a look at the entire code of your component?

Edwin Walela
1,373 PointsHere is the entire component;
import React from 'react';
import {
BrowserRouter,
Switch,
Route
} from 'react-router-dom';
//App Components
import Header from './Header';
import Home from './Home';
import About from './About';
import Courses from './Courses';
import Featured from './Featured';
import Teachers from './Teachers';
import NotFound from './NotFound';
const App = () => (
<BrowserRouter>
<div className="container">
<Header />
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" render={ ()=> <About title="About" /> } />
<Route path="/courses" component={Courses} />
<Route path="/featured" component={Featured} />
<Route exact path="/teachers" component={Teachers} />
<Route path="/teachers/:topic/:name" component={Featured} />
<Route component={NotFound} />
</Switch>
</div>
</BrowserRouter>
);
export default App;

Chris Underwood
Full Stack JavaScript Techdegree Student 19,916 PointsI am having the same issue with my application. I even copied the files for App.js, Home.js, and Featured.js. I actually needed to make a couple of changes to those files to get the files to even compile as I get an error that handleSubmit function is not defined.
/src/components/Home.js
Line 6: 'handleSubmit' is not defined no-undef
Specifically, I need to change the first line of handle submit from handleSubmit = (e) => {
to handleSubmit (e){
to get the code to compile. Then I need to change the onSubmit in the form from onSubmit={this.handleSubmit}
to onSubmit={e => this.handleSubmit}
to get the app to not crash when I submit. The enrror when I submit is
```TypeError: Cannot read property 'name' of undefined
handleSubmit
src/components/Home.js:8
5 |
6 | handleSubmit (e){
7 | e.preventDefault();
8 | let teacherName = this.name.value; 9 | let teacherTopic = this.topic.value; 10 | let path =
teachers/${teacherTopic}/${teacherName}
; 11 | this.props.history.push(path);```
With these extra features added the code runs but returns to the home page with the http://localhost:3000/? url described above.
App.js
import React from 'react';
import {BrowserRouter, Route, Switch} from 'react-router-dom';
//App components
import Header from "./Header";
import Home from "./Home";
import About from "./About";
import Teachers from "./Teachers";
import Courses from "./Courses";
import NotFound from "./NotFound";
import Featured from "./Featured";
const App = () => (
<BrowserRouter>
<div className="container">
<Header />
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" render={ () => <About title="About"/>} />
<Route exact path="/teachers" component={Teachers} />
<Route path="/teachers/:topic/:name" component={Featured} />
<Route path="/courses" component={Courses} />
<Route component={NotFound}/>
</Switch>
</div>
</BrowserRouter>
);
export default App;
Home.js
import React, { Component } from 'react';
class Home extends Component {
handleSubmit (e){
e.preventDefault();
let teacherName = this.name.value;
let teacherTopic = this.topic.value;
let path = `teachers/${teacherTopic}/${teacherName}`;
this.props.history.push(path);
}
render() {
return (
<div className="main-content home">
<h2>Front End Course Directory</h2>
<p>This fun directory is a project for the <em>React Router Basics</em> course on Treehouse.</p>
<p>Learn front end web development and much more! This simple directory app offers a preview of our course library. Choose from many hours of content, from HTML to CSS to JavaScript. Learn to code and get the skills you need to launch a new career in front end web development.</p>
<p>We have thousands of videos created by expert teachers on web design and front end development. Our library is continually refreshed with the latest on web technology so you will never fall behind.</p>
<hr />
<h3>Featured Teachers</h3>
<form onSubmit={e => this.handleSubmit}>
<input type="text" placeholder="Name" ref={ (input) => this.name = input } />
<input type="text" placeholder="Topic" ref={ (input) => this.topic = input } />
<button type="submit">Go!</button>
</form>
</div>
);
}
}
export default Home;
Featured.js
import React from 'react';
const Featured = ({match}) => {
let name = match.params.name;
let topic = match.params.topic;
return (
<div className="main-content">
<h2>{name} </h2>
<p>Introducing <strong>{name}</strong>, a teacher who loves teaching courses about <strong>{topic}</strong>!</p>
</div>
);
}
export default Featured;