Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial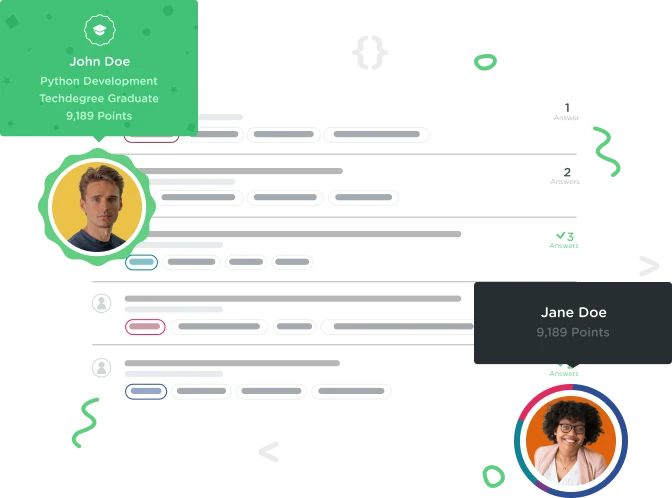
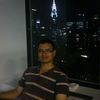
jerome sinpaseuth
2,632 Pointson the weather app i'm trying to change the color according to the temperature. any idea how to update the background ?
i did a if else to set new colors. but then i do not know how to update the color background according to these changes
6 Answers
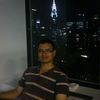
jerome sinpaseuth
2,632 Points<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".MainActivity" android:background="#16FEFF">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="--"
android:id="@+id/temperatureLabel"
android:layout_centerVertical="true"
android:layout_centerHorizontal="true"
android:textColor="@android:color/white"
android:textSize="150sp"/>
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/degreeImageView"
android:layout_alignTop="@+id/temperatureLabel"
android:layout_toRightOf="@+id/temperatureLabel"
android:layout_toEndOf="@+id/temperatureLabel"
android:src="@drawable/degree"
android:layout_marginTop="50dp"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="..."
android:id="@+id/timeLabel"
android:layout_centerHorizontal="true"
android:textColor="#80ffffff"
android:textSize="18sp"
android:layout_above="@+id/degreeImageView"/>
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/iconImageView"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:src="@drawable/cloudy_night"
android:layout_below="@+id/refreshImageView"
android:layout_alignRight="@+id/linearLayout"
android:layout_alignEnd="@+id/linearLayout"
android:layout_above="@+id/timeLabel"/>
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@+id/temperatureLabel"
android:layout_centerHorizontal="true"
android:layout_marginTop="10dp"
android:weightSum="100"
android:id="@+id/linearLayout">
<LinearLayout
android:orientation="vertical"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_weight="50">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="HUMIDITE"
android:id="@+id/humidityLabel"
android:textColor="#80ffffff"
android:gravity="center_horizontal"/>
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="--"
android:id="@+id/humidityValue"
android:textColor="@android:color/white"
android:textSize="24sp"
android:gravity="center_horizontal"/>
</LinearLayout>
<LinearLayout
android:orientation="vertical"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_weight="50">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="PLUIE /NEIGE ?"
android:id="@+id/precipLabel"
android:textColor="#80ffffff"
android:gravity="center_horizontal"/>
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="--"
android:id="@+id/precipValue"
android:textColor="@android:color/white"
android:textSize="24sp"
android:gravity="center_horizontal"/>
</LinearLayout>
</LinearLayout>
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/refreshImageView"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:src="@drawable/refresh"/>
<ProgressBar
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/progressBar"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_alignBottom="@+id/refreshImageView"/>
</RelativeLayout>
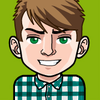
Harry James
14,780 PointsHey Jerome!
Interesting idea! It's great to see you going beyond what the course offers!
You can change the background of the LinearLayout with this piece of code:
linearLayout.setBackgroundColor(color)
You will need to replace linearLayout
with the variable name linking your LinearLayout and color
with your desired color :)
As for setting this color based on the temperature, I recommend you write the code to get the color in a separate class (It's just separation of concerns - it wouldn't make any difference as to how your app runs).
Then, in this new class (Which you could call ColorWheel
like in the Fun Facts app, for example) use some code like this:
MainClass mMainClass = new MainClass();
// Swap out MainClass for your main class (The user interface where the weather displays).
String mColor;
// We'll initialize our variable here so that we can use it below. You could also set a default value for it here and you wouldn't need the else condition below.
public String getBackgroundColor() {
if (mMainClass.temperature() > 100 && < 120) {
// Make a few if statements or use a case switch so that if the temperature is at a certain level, it goes a certain color.
mColor = "#FFFFFF";
// Set your color to the desired color.
}
else {
// This acts as a failsafe - if none of the statements above run, set the color to this:
mColor = "#000000";
// Set your color to the desired color.
}
return mColor;
}
Then, back in your code above, instead of using color
as the variable that sets the background color, you can swap it with mColorWheel.getBackgroundColor()
(Or rename ColorWheel if you picked a different name for your class, you will also need to instantiate the member variable with your class, like we did with the MainClass()
example above.
Hopefully this should make some sense but, if there's anything you don't understand, give me a shout :)
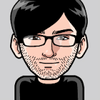
Alexandru Doru Grosu
2,753 PointsIf you are asking how to do this programatically, you will first have to retrieve the root layout. Assuming the root layout for my activity is a RelativeLayout
I would do it like this.
final RelativeLayout relativeLayout = (RelativeLayout) findViewById(R.id.idOfThisLayout);
And then to set the background color for this layout, I would
int yourColor = ...;
relativeLayout.setBackgroundColor(yourColor);
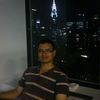
jerome sinpaseuth
2,632 Pointsthanks for the reply. i got some of it figured out. but since we're using butterknife api to update the content view, i'm a bit messed up. on my on create method i have:
setContentView(R.layout.activity_main); ButterKnife.inject(this);
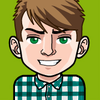
Harry James
14,780 PointsI haven't actually completed this course yet so wasn't aware of what this API did.
Could you provide your activity_main.xml
file here and I'll see what can be done?
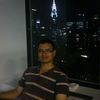
jerome sinpaseuth
2,632 Pointsi guess an easier way would be to make drawables for the background. and then change them but would be a bit unefficient.
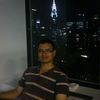
jerome sinpaseuth
2,632 Pointsclose but not quite there yet. the setbackground method does not work. i'll get back tomorow. late here in paris
Harry James
14,780 PointsHarry James
14,780 PointsAlright!
What you can do instead is wrap everything into a separate
RelativeLayout
orLinearLayout
(Either will do).RelativeLayout
So, wrap the code like this:
Then, just do the same as I specified above but with a RelativeLayout and everything should go fine :)
Or
LinearLayout
If you're wondering which one to pick, either will do - you won't notice any difference in this project, the only time you would possibly notice a difference is if you had a lot of views. You can read up more about the science of this on the internet if you're interested!
Hope it helps and, if you have any more questions, give me a shout :)