Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial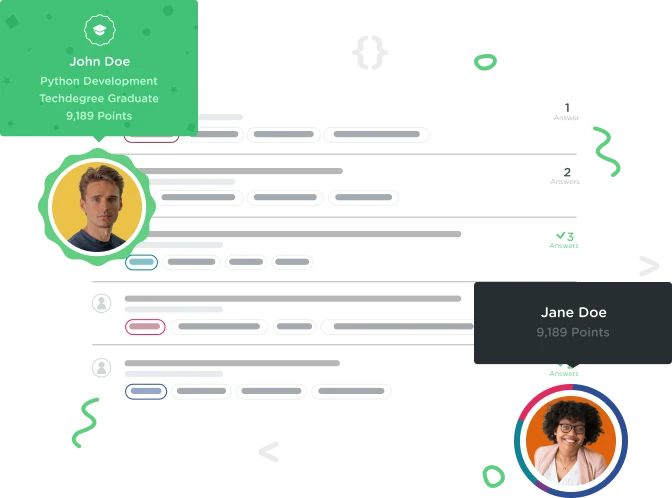

Harin Yuwarattanaporn
8,427 PointsOn worklog with database project, I have no idea what function to test? Can anyone give some example?
I'm finishing worklog with database using peewee. To pass, it require 50% coverage to test. I know how to test using unittest and doctest, But I don't know what function should I make a test in my worklog project? Almost every function i wrote must take action to .db file (such as add new entries, delete entries, search entries). I have no idea What to do and How to do test when getting involve with .db.?
1 Answer

Tonye Jack
Full Stack JavaScript Techdegree Student 12,469 PointsYou ca write the test cases for return values for select statements
import unittest
import models
class DatabaseTests(unittest.TestCase):
def setUp(self):
models.DATABASE.connect()
models.DATABASE.create_tables([models.User], safe=True)
def tearDown(self):
try:
models.DATABASE.drop_table(models.User)
except:
pass
models.DATABASE.close()
def test_check_user_table(self):
assert models.User.table_exists()
def test_safe(self):
with self.assertRaises(models.OperationalError):
models.DATABASE.create_table(models.User)
def test_drop_table(self):
models.DATABASE.drop_table(models.User)
assert models.User.table_exists() == False
class UserTableTests(unittest.TestCase):
def setUp(self):
models.DATABASE.connect()
try:
models.DATABASE.create_tables([models.User], safe=True)
except:
pass
models.User.create(
username='testUsername',
email='testEmail@testEmail.com')
models.DATABASE.close()
def tearDown(self):
models.User.delete().where(models.User.username=='testUsername').execute()
self.assertIsNone(models.User.select().where(models.User.username=='testUsername'))
def test_create_username(self):
user = models.User.get(username='testUsername')
self.assertEqual(user.username, 'testUsername')
def test_safe(self):
with self.assertRaises(models.IntegrityError):
models.User.create(
username='testUsername',
email='testEmail@testEmail.com')
if __name__ == '__main__':
unittest.main()