Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial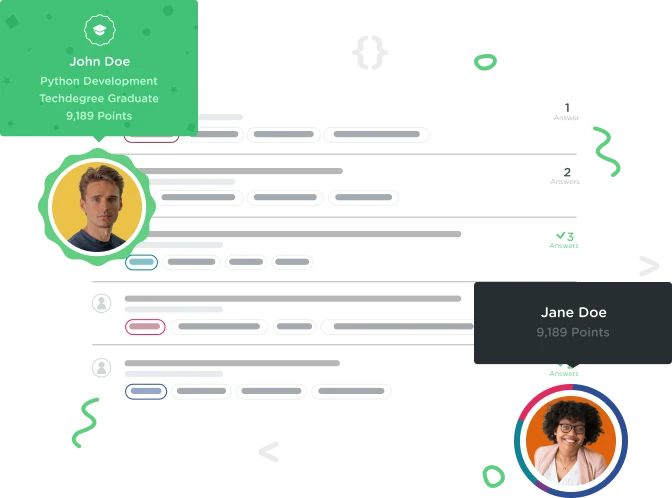
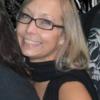
Tina Maddox
28,102 PointsOnce address was added contact names do not print. No errors.
Before I added the address.rb and the phone_number.rb the contact name; first_name, middle_name, last_name; would print. Now it won't but no errors. Like it isn't recognized.
Please help. I really do not like moving forward before I figure out what isn't working and my deadlines are pressing. Passing the code challenge is only part of this gig. :) Finding the mistakes is even better!
Thanks in advance!
require './phone_number'
require './address'
class Contact
attr_writer :first_name, :middle_name, :last_name
attr_reader :phone_numbers, :addresses
def initialize
@phone_numbers = []
@addresses = []
end
def add_phone_number(kind, number)
phone_number = PhoneNumber.new
phone_number.kind = kind
phone_number.number = number
phone_numbers.push(phone_number)
end
def add_address(kind, street_1, street_2, city, state, postal_code)
address = Address.new
address.kind = kind
address.street_1 = street_1
address.street_2 = street_2
address.city = city
address.state = state
address.postal_code = postal_code
addresses.push(address)
end
def first_name
@first_name
end
def middle_name
@middle_name
end
def last_name
@last_name
end
def first_last
first_name + " " + last_name
end
def last_first
last_first = last_name
last_first += ", "
last_first += first_name
if !@middle_name.nil?
last_first += " "
last_first += middle_name.slice(0, 1)
last_first += "."
end
last_first
end
def full_name
full_name = first_name
if !@middle_name.nil?
full_name += " "
full_name += middle_name
end
full_name += ' '
full_name += last_name
full_name
end
def to_s(format = 'full_name')
case format
when 'full_name'
full_name
when 'last_first'
last_first
when 'first'
first_name
when 'last'
last_name
else
first_last
end
end
def print_phone_numbers
puts "Phone Numbers"
phone_numbers.each { |phone_number| puts phone_number }
end
def print_addresses
puts "Addresses"
addresses.each { |address| puts address.to_s('short') }
end
end
jason = Contact.new
jason.first_name = "Jason"
jason.last_name = "Seifer"
jason.add_phone_number("Home", "123-456-7890")
jason.add_phone_number("Work", "456-789-0123")
jason.add_address("Home", "123 Main St.", "", "Portland", "OR", "12345")
puts jason.to_s('full_name')
jason.print_phone_numbers
jason.print_addresses
class Address
attr_accessor :kind, :street_1, :street_2, :city, :state, :postal_code
def to_s(format = 'short')
address = ''
case format
when 'long'
address += street_1 + "\n"
address += street_2 + "\n" if !street_2.nil?
address += "#{city}, #{state} #{postal_code}"
when 'short'
address += "#{kind}: "
address += street_1
if street_2
address += " " + street_2
end
address += ", #{city}, #{state} #{postal_code}"
end
address
end
end
home = Address.new
home.kind = "Home"
home.street_1 = "123 Main St."
home.city = "Portland"
home.state = "OR"
home.postal_code = "12345"
puts home.to_s('short')
puts "\n"
puts home.to_s('long')
class PhoneNumber
attr_accessor :kind, :number
def to_s
"#{kind}: #{number}"
end
end
2 Answers

Kirill Lofichenko
10,761 PointsThe issue could be in your "require" statements at the very top of the contact.rb file They do not describe the proper path to the files, both are missing the file extension piece ".rb". So chances are the files are not actually being loaded for use. I'd start with checking there.

Kirill Lofichenko
10,761 PointsNo problem. Good luck with the rest of the project!
Tina Maddox
28,102 PointsTina Maddox
28,102 PointsHello Kirill, Thank you so much! You were right. As soon as I read your post... I knew you were right!
It amazes me how easy it is to miss the little things... that break the code.
Thanks you again!! Blessings, Tina