Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial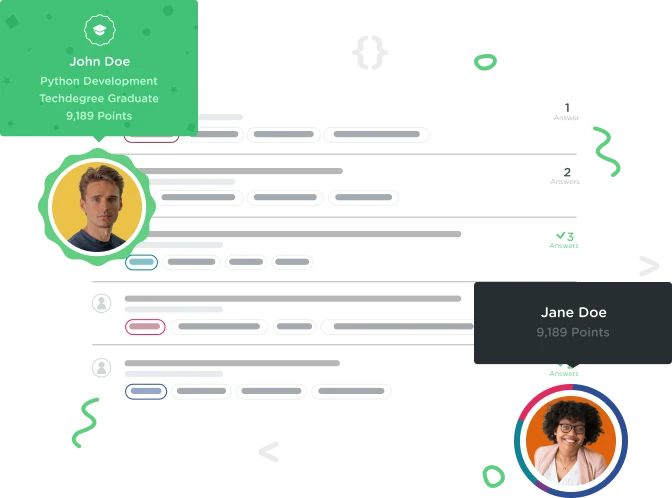
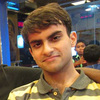
Divyansh Upreti
2,011 PointsonClickListener problem.
Hi guys, i am always scared by this code which is used very often... can someone explain this completely in a nice way to me...
What are the parameters of the method and then there is a method within a method... its all confusing...
mGetAnswerButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View arg0) {
// TODO Auto-generated method stub
}
});
1 Answer
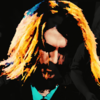
Justin Horner
Treehouse Guest TeacherHello Divyansh,
I'll certainly do my best to explain what's going on.
Firstly, the button needs a callback, some code to call when the button gets clicked. The way we set that callback is with the setOnClickListener.
Looking at the documentation for setOnClickListener, we can see that it takes an implementer View.OnClickListener as a parameter.
[Documentation - setOnClickListener](http://developer.android.com/reference/android/view/View.html#setOnClickListener(android.view.View.OnClickListener)
So what is View.OnClickListener? From the documentation it an Interface definition for a callback to be invoked when a view is clicked.
Documentation - View.OnClickListener
So we know we need to pass an instance of an object that implements the View.OnClickListener interface. If the class you're working in doesn't implement View.OnClickListener than the common way to accomplish this is to create an anonymous inner class.
Now here is where the magic happens. Let's look at the button click example again.
mGetAnswerButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View arg0) {
// TODO Auto-generated method stub
}
});
Here's the breakdown of what's happening here. By creating a new View.OnClickListener() and defining the code block, you are essentially creating an anonymous inner class that implements View.OnClickListener.
This is the same net result of creating a new Java class that implements View.OnClickListener, creating an instance of it and passing it to the setOnClickListener method.
I hope this helps. If you still have questions I'll do my best to explain further.
Divyansh Upreti
2,011 PointsDivyansh Upreti
2,011 PointsThanks for answering Justin! I have got some doubts...
the button 'mGetAnswerButton' is itself a view... and we are calling setOnClickListener on it, then why does it need a new View as a parameter? is this just a convention?
if i don't create the 'anonymous inner class' and rather create it separately in my code, override the onClick method and then call its instance as my parameter in my setOnClickListener method instead, it should work... isn't it? it's just that the above code looks too ugly to look at.
Justin Horner
Treehouse Guest TeacherJustin Horner
Treehouse Guest TeacherYou're welcome! About the other questions...
Remember that setOnClickListener does not necessarily take a view as a parameter. it takes a class that implements the View.OnClickListener interface. This class being passed in implements the interface which means when the button is clicked, the framework can trust that an onClick method exists on the object is passed to it as the callback.
Yes, in fact there are several approaches to setting button click listeners. Check out the documentation for Button. In the class overview, second example you'll see you can also define them in xml and simply have a matching method in your activity that has a View as a parameter. The view parameter is simply a way to get the sender, or the button clicked, to the callback.
Another approach would be to make your Activity class implement View.OnClickListener and use a switch on the view id to find which button was clicked like this.
So you can see in this example when calling setOnClickListener we're passing 'this' as the View.OnClickListener because our Activity class implements the View.OnClickListener interface.
I hope this helps.
Divyansh Upreti
2,011 PointsDivyansh Upreti
2,011 PointsThanks Justin!
Justin Horner
Treehouse Guest TeacherJustin Horner
Treehouse Guest TeacherYou're welcome!