Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial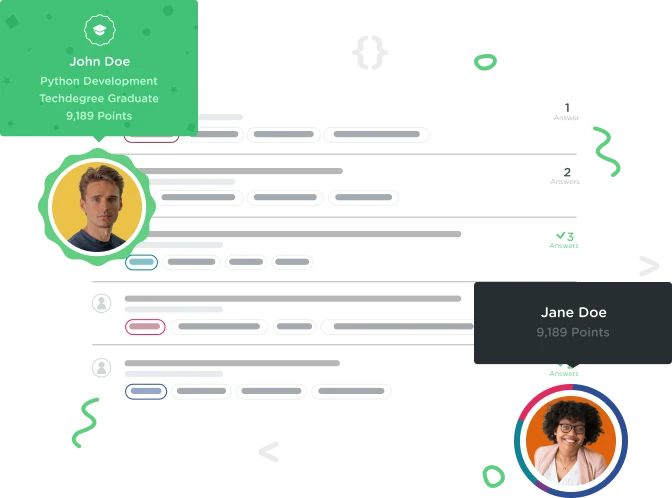

Kevin Ohlsson
4,559 Pointsone solution
here is code for solution, have to use quit to show result, searches through people with same name. Can alert that no people in database with name via console.log but current implementation is buggy. This code should be refactored.
let students = [
{
name: "Kevin",
track: "JavaScript",
achievements: "123",
points: 3500,
},
{
name: "Joe",
track: "LUA",
achievements: "312",
points: 2121
},
{
name: "Anita",
track: "haskell",
achievements: "All",
points: 3000
},
{
name: "Siv",
track: "completed everything",
achievements: "All",
points: 99999
},
{
name: "Olle",
track: "completed everything",
achievements: "All",
points: 99999
},
]
function div(arg) {
document.write(`<div>${arg}</div>`)
}
function h1(arg) {
return `<h1>${arg}</h1>`
}
function paragraph(arg) {
return `<p>${arg}</p>`
}
while (true) {
let search = prompt("Search for students. Type 'q' to exit. Type 'l' to list all students.")
let searchWash = search.toLowerCase()
if (search === "q") {
break;
}
searchStudent(searchWash)
}
//go thorough the list that is the students
function searchStudent(name) {
for (let i = 0; i < students.length; i++) {
let stuObj = students[i];
// console.log(stuObj)
let studentName = stuObj.name;
studentName = studentName.toLowerCase()
if (name === studentName) {
console.log(`"${name}" found in database`)
allProps = h1(studentName) + paragraph(`Track : ${stuObj.track}`) + paragraph(`Achievements : ${stuObj.achievements}`) + paragraph(`Points: ${stuObj.points}`) // wrap all properties using functions
div(allProps) //wrap in div and print using document.write
} else {
console.log(`student "${name}" not in database`);
}
}
}
1 Answer
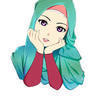
Karina Gubaidullina
Courses Plus Student 8,318 PointsAnd this is my solution. Based on the knowledge from courses 'JavaScript Basics' and 'JavaScript Loops, Arrays and Objects' :
var input;
var student;
var studentName;
var message = '';
var noStudent = '';
var counter = 0;
var i = 0;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
while (true) {
input = prompt('Type the name of student or type \"quit\" to cancel');
counter = 0;
i = 0;
if ( input.toUpperCase() === 'QUIT' || input === null ) {
break;
}
while ( i < students.length ) {
student = students[i];
studentName = student.name;
if ( input.toUpperCase() === studentName.toUpperCase() ){
for ( var key in student ) {
if ( key === 'name' ) {
message += '<h2>Student: ' + studentName + '</h2>';
} else {
message += '<p>'+ key + ': ' + student[key];
}
}
} else {
counter += 1;
if ( counter === students.length ) {
message += '<h2>No student with the name ' + input + '</h2>';
}
}
print(message);
i += 1;
}
}