Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial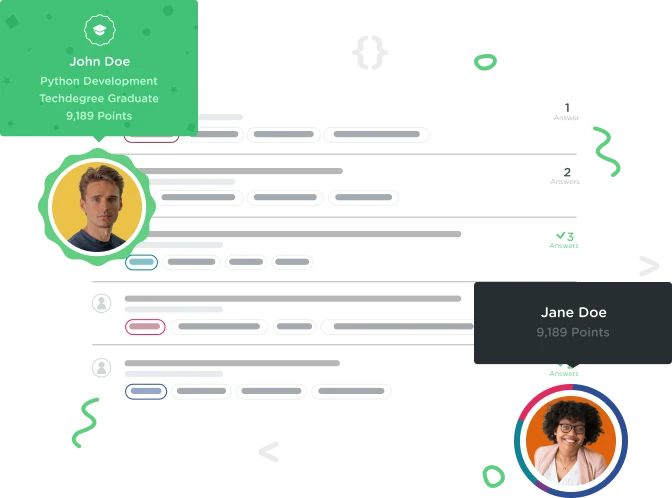
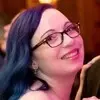
lythila
2,958 PointsOne step further - hiding the remove button when there are no more items
Hello!
I wanted to test my learnings and go one step further and hide the remove task button once there were no more items in the list and then reshow it once an item was added.
Based on the information from this track does this seem like an efficient solution or is there a better way to do this?
const btnCreate = document.querySelector('.btn-main');
const btnToggle = document.querySelector('.btn-toggle');
const btnRemove = document.querySelector('.btn-remove');
const elements = document.querySelector('ul').getElementsByTagName('li');
btnCreate.addEventListener('click', () => {
const input = document.querySelector('.input-main');
const list = document.querySelector('ul');
list.insertAdjacentHTML(
'afterbegin',
`<li>${input.value}</li>`
);
input.value = '';
if (btnRemove.style.display === 'none') {
btnRemove.style.display = 'block';
}
});
btnToggle.addEventListener('click', () => {
const listContainer = document.querySelector('.list-container');
if (listContainer.style.display === 'none') {
btnToggle.textContent = 'Hide List';
listContainer.removeAttribute('style');
} else {
btnToggle.textContent = 'Show List';
listContainer.style.display = 'none';
}
});
btnRemove.addEventListener('click', () => {
const lastItem = document.querySelector('li:last-child');
lastItem.remove();
if (elements.length === 0) {
btnRemove.style.display = 'none';
}
});
2 Answers
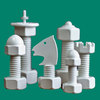
Steven Parker
231,236 PointsIt seems a bit awkward to handle button display different ways at different times. So instead of completely removing the "style" attribute in one handler, you might just set style.display to "block" as is done in the other handler.
This can lead to a small efficiency improvement, as you won't have to test if it is already "none" when you want it to be "block".
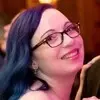
lythila
2,958 PointsOhhh I see what you're saying! That makes a lot of sense. Thanks for the input!!