Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial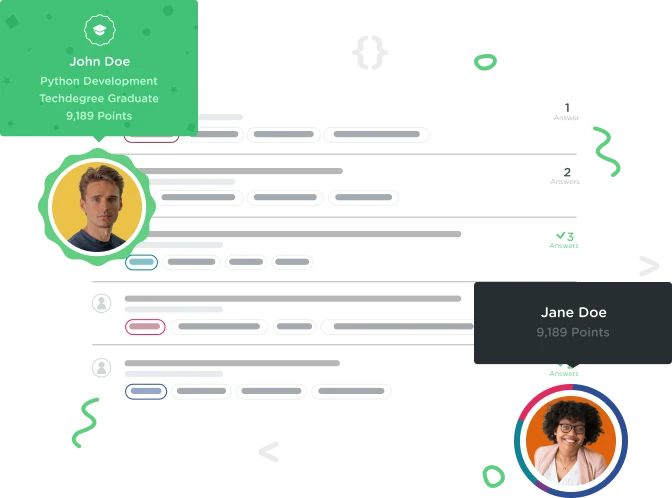
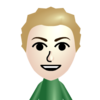
Daniel Granger
Courses Plus Student 19,857 Points.on("error", function(){...}); Not catching Error event.
My code is not catching the error event, instead it just shows:
_http_client.js:25
throw new Error('Unable to determine the domain name');
^
Error: Unable to determine the domain name
at new ClientRequest (_http_client.js:25:13)
at Object.exports.request (http.js:31:10)
at Object.exports.get (http.js:35:21)
at Object.<anonymous> (/Users/d/Sites/sandbox/JavaScript/Node/treehouseApp.js:13:20)
at Module._compile (module.js:413:34)
at Object.Module._extensions..js (module.js:422:10)
at Module.load (module.js:357:32)
at Function.Module._load (module.js:314:12)
at Function.Module.runMain (module.js:447:10)
at startup (node.js:140:18)
Here is my code:
// Connect to the API URL (http://teamtreehouse/username.json)
var request = http.get("teamtreehouse.com/" + username + ".json", function(response){
console.log(response.statusCode);
// Read the data
// Parse the data
// Print the data
});
request.on("error", function(e){
console.error(e.message);
});
As per the instructions in the video, the protocol in the URL has been omitted to ensure an error is thrown, however the error event is not caught and thus console.error(e.message);
is not shown.
I'm using Node.js 5.6.0.
Thanks
4 Answers
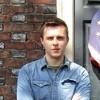
Kirill Babkin
19,940 Pointsi did this.
var https = require("https")
// print message
try{
var request = https.get("teamtreehouse.com/username.json",function(response){
console.log(response.statusCode); // print the data
});
} catch(e) {
console.error(e.message);
}
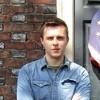
Kirill Babkin
19,940 Pointstry{ }catch(error){ } block works on all errors. request.on("error", function(e){ console.error(e.message); }); may not work depending on the version of node.
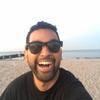
Anthony Albertorio
22,624 PointsGreat suggestion. Works like a charm. This video needs updating to avoid confusion. Thanks!
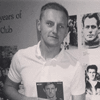
Dom Farnham
19,421 PointsThanks

Sebastian Röder
13,878 PointsA better way to simulate the error
event is keep the correct URL intact:
"http://teamtreehouse.com/" + username + ".json"
and instead disconnect your internet connection temporarily while you execute node app.js
. This will result in the following error event:
$ node app.js
events.js:154
throw er; // Unhandled 'error' event
^
Error: getaddrinfo ENOTFOUND teamtreehouse.com teamtreehouse.com:443
at errnoException (dns.js:26:10)
at GetAddrInfoReqWrap.onlookup [as oncomplete] (dns.js:77:26)
If you handle the error
event in the callback, as described in the video, the error message will look like this instead:
$ node app.js
getaddrinfo ENOTFOUND teamtreehouse.com teamtreehouse.com:443
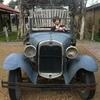
Brendan Moran
14,052 PointsThe documentation is a bit confusing, too. I tried exactly what it says in the documentation for an HTTPS request error:
const https = require('https');
https.get('https://encrypted.google.com/', (res) => {
console.log('statusCode: ', res.statusCode);
console.log('headers: ', res.headers);
res.on('data', (d) => {
process.stdout.write(d);
});
}).on('error', (e) => {
console.error(e);
});
Notice that the error handler is on the response, not the request. I found this confusing, because in the HTTP documentation, it is on the request, like this:
http.get('http://www.google.com/index.html', (res) => {
console.log(`Got response: ${res.statusCode}`);
// consume response body
res.resume();
}).on('error', (e) => {
console.log(`Got error: ${e.message}`);
});
I tried both, and Andrew's way. None of it worked, so that leaves me confused as to why the currently documented way does't work, either. "node- v" command is showing 6.2.2 for me in the workspaces console. The try/catch method did work, though, so thank you Kirill Babkin for that.
Chris Skinner
3,807 PointsChris Skinner
3,807 PointsSo what does this mean in terms of forcing / caching an error for demonstration purposes - is there a work around / solution\?
Dom Farnham
19,421 PointsDom Farnham
19,421 PointsGood to know. Thanks
Manav Misra
11,778 PointsManav Misra
11,778 PointsMore specifically, using this worked:
var request = https.get({host:"teamtreehouse.com/" + username + ".json"}, function(response){
http://stackoverflow.com/questions/32675907/how-to-deal-with-unhandled-error-event-error-nodejs