Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial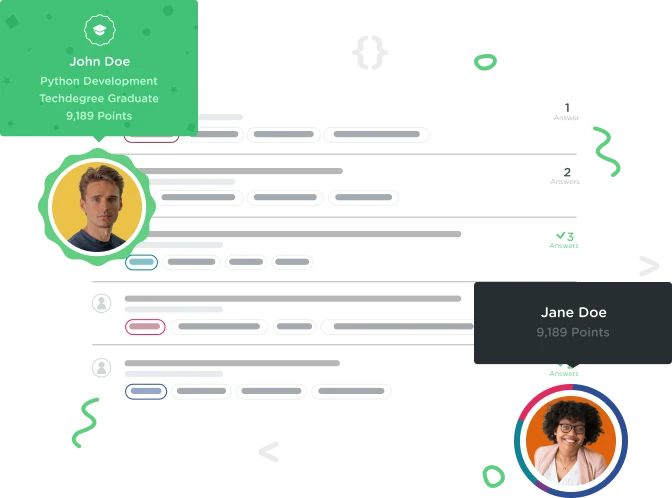

Judith Straetemans
1,807 PointsOnly 2 quotes are being accessed from Array with 10 quotes
import UIKit
class ViewController: UIViewController {
@IBOutlet weak var funFactLabel: UILabel!
let factBook = FactBook()
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
funFactLabel.text = factBook.factsArray[0]
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
@IBAction func showFunFact() {
funFactLabel.text = factBook.factsArray[1]
}
}
When I run this code in the simulator it only shows 2 quotes, where I have 10 in the Array. How come?
3 Answers
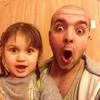
Ghaith Ali
3,134 PointsHey Judith, the problem in your code is that you are only accessing the first and the second element in the array, you show the first element in the viewDidLoad() function and you show the second when you call the showFunFact() function. You need a way to show the next element in the array, what I would suggest is making a counter, and then incrementing it every time you call the showFunFact(), like the following:
class ViewController: UIViewController {
@IBOutlet weak var funFactLabel: UILabel!
let counter : Int = 0
let factBook = FactBook()
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
funFactLabel.text = factBook.factsArray[0]
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
@IBAction func showFunFact() {
funFactLabel.text = factBook.factsArray[counter]
if counter < factBook.factsArray.count-1 {
counter++
} else {
counter = 0
}
}
}
what we are doing here is adding an if statement, if the counter value is less than the number of elements in the array, then increment the counter by 1 and show the next element in the array, if the counter value equal or more than the number of elements in the array, then reset the counter to 0 to show back the first element. Later in the lessons you will learn how to make a random fact show up. I hope my answer makes sense, ask again if you need more help.
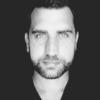
Jhoan Arango
14,575 PointsHello:
From what I can see you have hard coded to only show two of the 10 quotes.
The first one that shows, is the one from when the viewDidLoad()method is executed, and the other one, which is the one you show when the showFunFact() action (button is pressed) is executed.
I am not sure how far along you are in the course, but I am sure the answer you are looking for is near ahead in the videos. From what I remember in the course, he first shows you how to get the quotes, and then he creates a random quote generator.
Hang tight, the answer is coming ahead.
Good luck.

Judith Straetemans
1,807 PointsThanks for the answers!
I was already thinking: why do I not need to increment this, but I copied the code from the instruction video. I guess it's confusing that they ask you to build something but don't explain everything until later on.