Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial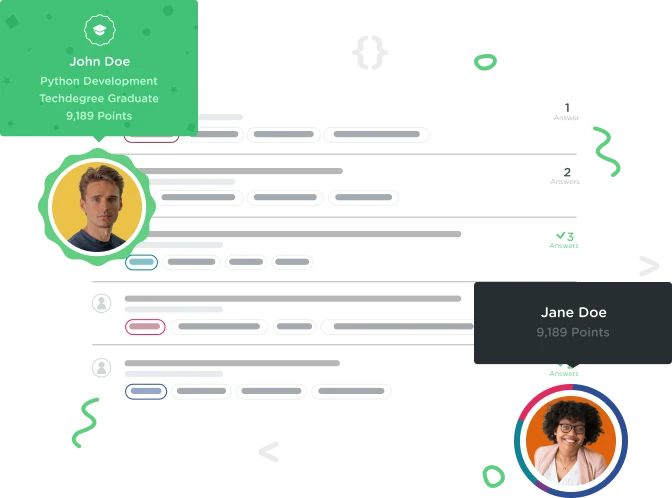

Venkiteswaran Subramanian
7,643 PointsOnly odd indexed items in the list getting deleted while trying to clear the entire list using remove method
I am trying to clear all the items in a list using remove() function using the below code. But, only the odd numbered items are getting removed from the list
code:
def clear_items(): for item in shopping_list: shopping_list.remove(item)
4 Answers
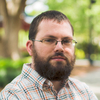
Kenneth Love
Treehouse Guest TeacherDon't modify the length of a list while you're working through it. If you need to zero out the list for some reason, either set it to a new, blank list:
my_list = []
Or use the .clear()
method.
my_list.clear()

Geroge Harris
1,279 Pointstried below and it worked (put a while loop before the for iteration)
def clear_items(list_to_clear): while len(list_to_clear)>0: for item in list_to_clear: list_to_clear.remove(item)
clear_items(shopping_list)
you could also just use shopping_list =0 if you don't have to use remove.

Venkiteswaran Subramanian
7,643 PointsThanks everyone! Answers were really helpful.
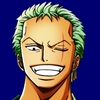
David Sudermann
Courses Plus Student 3,228 PointsIt's an old question, but since I ran into the very same problem, here my solution:
def clear_items(a_list):
for item in a_list[:]:
a_list.remove(item)
This way the list that is being iterated over is not changing and skipping issues are prevented.
For clearing purposes setting the list to an empty list, like Kenneth suggested, is more efficient. I used it to remove non-integer items:
def remove_nonint(a_list):
for item in a_list[:]:
if type(item) != int:
a_list.remove(item)
Thomas Kirk
5,246 PointsThomas Kirk
5,246 PointsAs I understand it, your for loop is removing an item from each index point.
It removes the item at shopping_list[0], then the item at shopping_list[1],...
The problem with this is, as items are removed, the index of the remaining items change. When [0] is removed, [1] becomes [0], and [2] becomes [1] etc, so items are skipped in the loop.