Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial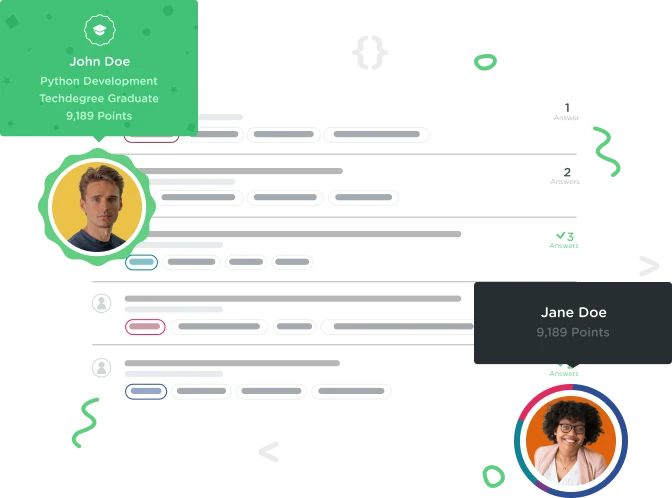
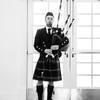
Scott Pileggi
Courses Plus Student 430 PointsOnly receiving output from one of the two 'Value Errors' whether I enter text instead of int, or an int > tickets_left
Hello everyone,
I've been really happy with my progress this week. But I'm running into a bit of confusion at this point of the project. Let me start by sharing my code:
TICKET_PRICE = 10
tickets_remaining = 100
while int(tickets_remaining) >= 1:
print("There are {} tickets remaining".format(tickets_remaining))
first_name = input("What is your first name? ")
number_of_tickets = input("Hello {}. How many tickets would you like? ".format(first_name))
#Expect a ValueError to happen and handle it appropriately...remember to test it out
try:
number_of_tickets = int(number_of_tickets)
# Raise a ValueError if the request is for more tickets than are available.
if number_of_tickets > tickets_remaining:
raise ValueError("There are only {} tickets remaining.".format(tickets_remaining))
except ValueError as err:
# Include the error text in the output
print("Oh no! Number of tickets must be numeric... Try again")
else:
total_cost = (number_of_tickets * TICKET_PRICE)
print("Amount due is ${}".format(total_cost))
confirmation = input("Do you want to proceed? Y/N ")
if confirmation.lower() == ("y"):
print("SOLD!")
tickets_remaining = tickets_remaining - number_of_tickets
else:
print("Thank you anyways, {}".format(first_name))
print("Sorry. Tickets for this show are sold out. :(")
There are no ugly error messages that pop up while this code runs. So that's a step in the right direction. However, it seems that only one of the two ValueErrors pops up not only if I enter a non-integer (which is correct). But I also get the same Value Error printed back to the user if the amount of tickets desired is greater than the amount of tickets available.
Take a look here:
treehouse:~/workspace$ python masterticket.py
There are 100 tickets remaining
What is your first name? Scott
Hello Scott. How many tickets would you like? blue
Oh no! Number of tickets must be numeric... Try again
There are 100 tickets remaining
What is your first name? Scott
Hello Scott. How many tickets would you like? 1000
Oh no! Number of tickets must be numeric... Try again
There are 100 tickets remaining
What is your first name?
Basically, I'm just having trouble getting the 'greater than' exception to work correctly. Any help is greatly appreciated.
Thank you! Scott
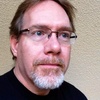
Chris Freeman
Treehouse Moderator 68,441 PointsThe colorized format comes from adding python after the backticks:
```python
2 Answers
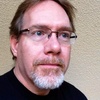
Chris Freeman
Treehouse Moderator 68,441 PointsIt’s not a formatting issue. The error you’re seeing is the actual output from the ValueError
>>> int('blue')
Traceback (most recent call last):
File “<stdin>”, line 1, in <module>
ValueError: invalid literal for int() with base 10: ‘blue’
The trouble with using as err
Is your stuck with whatever the system puts out.
Post back if you need more help. Good luck!!!
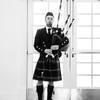
Scott Pileggi
Courses Plus Student 430 PointsChris, thank you for always being such a timely and helpful resource!!
appreciate the answers to both of my questions :)
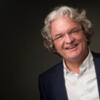
Dirk Burger
314 PointsHi Chris, thank you for your explantion. However what is the appropriate solution to avoid customer seeing "whatever the system puts out"?
Thank you.
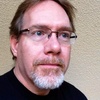
Chris Freeman
Treehouse Moderator 68,441 PointsIf we ignore that point of the challenge is to learn how to pass strings into except
code blocks, to avoid the system error output stings, leave off the as err
and use print statements. Sometimes might involve restructuring the code to get the desired flow, or encapsulated the too large ticket request in its own try block.
In this case, since the flow is fall to the bottom of the while
loop, the following might suffice:
try:
number_of_tickets = int(number_of_tickets)
# Indicate error if the request is for more tickets than are available.
if number_of_tickets > tickets_remaining:
print("There are only {} tickets remaining. Please try again.".format(tickets_remaining))
# jump to top of while loop
continue
except ValueError:
# Include the error text in the output
print("Oh no, we ran into an issue. Number of tickets must be an integer. Please try again.")
Scott Pileggi
Courses Plus Student 430 PointsScott Pileggi
Courses Plus Student 430 PointsSo I have managed to get my first question sorted out. My code just has one issue left. When my application asks "How many tickets would you like?" if I respond with a non-integer like "blue", I get some sort of formatting issue. Let me post my most up to date exception code:
So when I use the application based on the exceptions above and I input something like "blue" for the ticket amount, I get the following output; which is partially correct:
'Oh no, we ran into an issue. invalid literal for int() with base 10: 'greg'. Please try again'
Clearly a formatting issue. Once again, any input would be appreciated.
I'm actually really stoked with how far I've come over a couple of days. Been trying to drill these concepts into my mind because I'm starting to get quite eager to excel at this tech degree program.
Oh, and one more question. I know you surround your code with (```) to share it on a forum. But I noticed mine is all white text. How do I make it syntax color like you all do?