Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial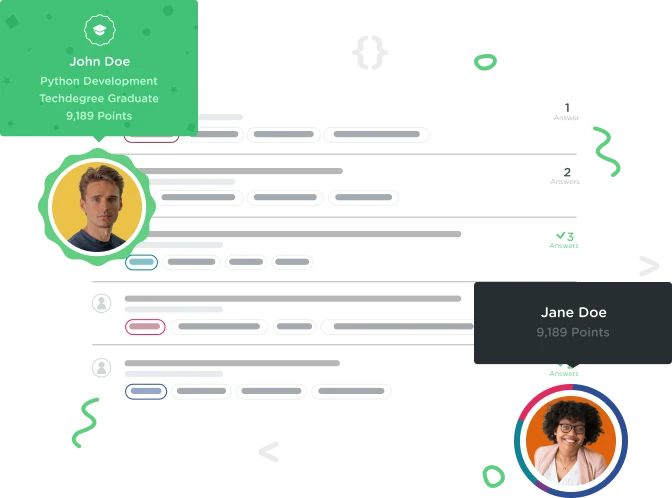

Yang Bi
10,079 Pointsonly removed half of the vowels..
ok. here is my script for this disemvowel question:
def disemvowel(word):
word = list(word)
for letter in word:
if letter == 'a':
word.remove('a')
elif letter == 'e':
word.remove('e')
elif letter == 'i':
word.remove('i')
elif letter == 'o':
word.remove('o')
elif letter == 'u':
word.remove('u')
elif letter == 'A':
word.remove('A')
elif letter == 'E':
word.remove('E')
elif letter == 'I':
word.remove('I')
elif letter == 'O':
word.remove('O')
elif letter == 'U':
word.remove('U')
word = ''.join(word)
return word
print (disemvowel("aaaaaaeeeeeeiiiiiioooooouuuuuuf"))
running this script I can only remove half of the vowels. so my result is "aaaeeeiiiooouuuf" .
I don't know why this happened. is it because of the for loop is not looping every letter in my word? or my conditional if loop is skipping once every two vowels?
[MOD: added ```python formatting -cf]
2 Answers
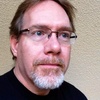
Chris Freeman
Treehouse Moderator 68,423 PointsThere are two issues:
-
the argument
word
is a string which does not have aremove()
method. You will need to convert to a list first:word = list(word)
Remember to convert the list back to a string using
"".join(word)
-
As Steven mention, you shouldn't modify the iterable of a for loop. Use a copy instead:
# use copy(): for letter in word.copy(): # use a slice (you will learn about this later in the Python course: for letter in word[:]:
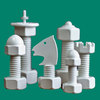
Steven Parker
230,274 PointsNever modify an iterable within the loop it is controllling, this can cause items to be skipped over.
Instead, iterate using a copy of the item, or construct a new item to return in the loop instead of modifying the original.
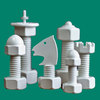
Steven Parker
230,274 PointsI forgot to mention the point Chris pointed out about strings not having a remove method. But, if you wanted to use a slightly different strategy, they do have a replace method.

Yang Bi
10,079 PointsThank you Steven for your quick response.
Yang Bi
10,079 PointsYang Bi
10,079 PointsThanks for the demo code. :)