Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial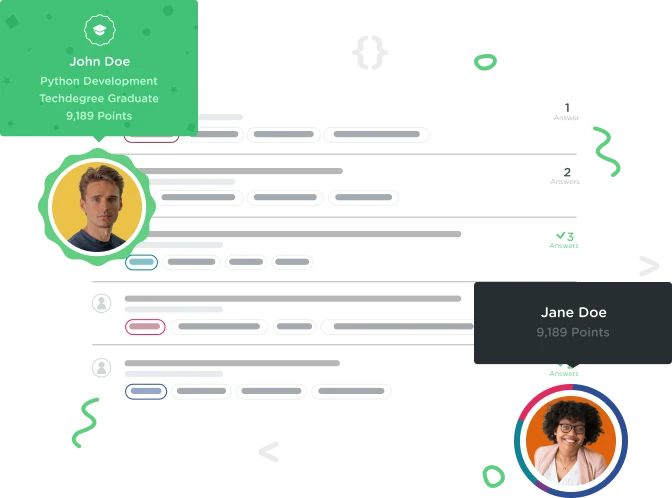
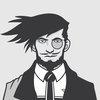
edinjusupovic
5,664 PointsOnly the logo fades in, all other images load abnormally
My code is as follows
HTML CODE
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Treehouse Pet Adoption</title>
<link rel="shortcut icon" href="favicon.ico" type="image/x-icon">
<link rel="icon" href="favicon.ico" type="image/x-icon">
<link href="https://fonts.googleapis.com/css?family=Bree+Serif|Open+Sans:400,700" rel="stylesheet">
<link rel="stylesheet" href="styles.css">
</head>
<body>
<header>
<nav class="container">
<h1 class="logo"><img src="images/logo.svg" /></h1>
<ul>
<li><a href="index.html">Adopt</a></li>
<li><a href="post.html">Post</a></li>
</ul>
</nav>
</header>
<h2 class="page-header">These puppies want to find their furever homes!</h2>
<main class="container adoptable">
<div class="row">
<section class="six columns">
<div class="card">
<img src="images/puppy1.jpg" alt="french bulldog puppy">
<p>
<strong>Name:</strong> Florence
</p>
<p class="loc">
<strong>Location:</strong> Treehouse Adoption Center
</p>
<p>
<strong>Notes:</strong> Great at rubber duck debugging!
</p>
<button class="full">Adopt!</button>
</div>
</section>
<section class="six columns">
<div class="card">
<img src="images/puppy2.jpg" alt="french bulldog puppy">
<p>
<strong>Name:</strong> Henrietta
</p>
<p class="loc">
<strong>Location:</strong> Treehouse Adoption Center
</p>
<p>
<strong>Notes:</strong> Gets a little frisky around Easter candy.
</p>
<button class="full">Adopt!</button>
</div>
</section>
</div>
<div class="row">
<section class="six columns">
<div class="card">
<img src="images/puppy3.jpg" alt="french bulldog puppy">
<p>
<strong>Name:</strong> Jerry
</p>
<p class="loc">
<strong>Location:</strong> Treehouse Adoption Center
</p>
<p>
<strong>Notes:</strong> Can use his ears as wings.
</p>
<button class="full">Adopt!</button>
</div>
</section>
<section class="six columns">
<div class="card">
<img src="images/puppy4.jpg" alt="french bulldog puppy">
<p>
<strong>Name:</strong> Norma
</p>
<p class="loc">
<strong>Location:</strong> Treehouse Adoption Center
</p>
<p>
<strong>Notes:</strong> Loves a good bath.
</p>
<button class="full">Adopt!</button>
</div>
</section>
</div>
</main>
<footer>
<p class="container">
Made with <3 fur puppies by <a href="#">Aisha Blake</a>
</p>
</footer>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.0.0/jquery.min.js"></script>
<script src="app.js"></script>
</body>
</html>
JS CODE
$('.loc').hover(
function(){
$(this).html("<strong>Location:</strong> Your house?!");
},
function() {
$(this).html("<strong>Location:</strong> Treehouse Adoption Center");
});
// Grab info from the form
$('#add-pet').on('click', function() {
var $name = $('#pet-name').val();
var $species = $('#pet-species').val();
var $notes = $('#pet-notes').val();
// Assemble the HTML of our new elemen with the above variables
var $newPet = $(
'<section class="six columns"><div class="card"><p><strong>Name:</strong> ' + $name +
'</p><p><strong>Species:</strong> ' + $species +
'</p><p><strong>Notes:</strong> ' + $notes +
'</p><span class="close">×</span></div></section>'
);
// Attach the new element to the page
$('#posted-pets').append($newPet);
});
$('img').css('display', 'none').fadeIn(1600);
Tested browser is chrome. When the site is run only the logo fades in as expected, the images of the puppies all load abnormally - ie; it appears they don't load for 1.6 seconds and then load instantly instead of fading in as expected - the code is all copied from the video so I'm not quite sure what the issue is.
3 Answers
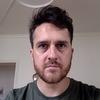
Raphaël Seguin
Full Stack JavaScript Techdegree Graduate 29,228 PointsMaybe, you should try encapsulating your script into an "onlad" function to launch it after all the assets are downloaded, like this :
window.onload = function() {
//your code here
}
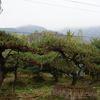
Learning coding
Front End Web Development Techdegree Student 9,937 PointsAround the scripts in the html markup? That's what i tried, but it didn't work. I also wrapped this code around: $('img').css('display', 'none').fadeIn(1600); Both ways didn't work.
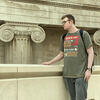
Stan Day
36,802 PointsHow fast is your internet? Most likely the images are just loading very slow so during the fade in step they are not loaded and you can't see anything and then when they load it looks sudden.
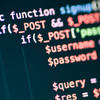
Justin Radcliffe
18,987 PointsAfter reviewing the answers here, none of them addressed my problem successfully, so after reviewing the code in the master zip file for this project I identified that the code is a little different:
// Puppy images fade in
$('main img').css('display', 'none').fadeIn(1600);
This fixed my problem.
Learning coding
Front End Web Development Techdegree Student 9,937 PointsLearning coding
Front End Web Development Techdegree Student 9,937 PointsSame issue here.
Only the logo fades in, the images load in abnormally. https://w.trhou.se/5igznfpcqw