Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial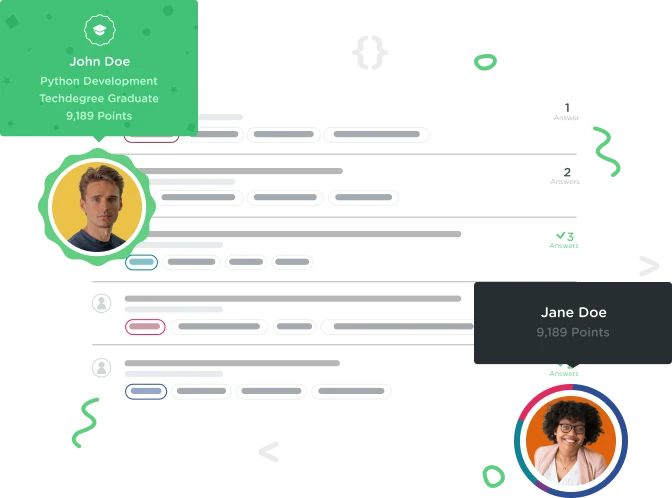

Jassim Alhatem
20,883 PointsOOP. Magic Methods.
So I just started a new "level" in OOP. And learnt something called magic methods. Sadly, I didn't really understand much of the video.
Can someone explain to me what (Dunder)int(Dunder) and (Dunder)str(Dunder) do? And how do I call in a class from a different file?
Thank you!
1 Answer
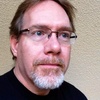
Chris Freeman
Treehouse Moderator 68,423 PointsGreat question! According to PEP08 Name Conventions,
"Magic" methods, sometimes called "dunder" methods due to the leading and trailing double_underscore (__
) in their names, are "magic" objects or attributes that live in user-controlled namespaces. E.g. __init__
, __import__
or __file__
. Never invent such names; only use them as documented.
The magic methods provide ways for the built-in functions to operate on objects. For example, getting a string representation of an object to print, converting to an integer for math computations, comparing objects to one another, etc.
Some classes define additional dunder methods that are accessed by specific built in functions. Using the built-in function str(some_object)
tries to convert some_object into a string, The str()
function tries to call the some_object.__str__()
method to see if some_object
has defined a string representation of itself. Similarly, the built-in function int(another_object)
would call the another_object.__int__()
method to get help from the object to convert the object into an integer. Without the "dunder it" method present, calling int()
on an object could raise an error:
# Define an empty class
>>> class Foo:
... pass
...
# create instance and covert to integer
>>> int(Foo())
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: int() argument must be a string, a bytes-like object or a number, not 'foo'
# Redefine class to have an `__int__` method, that always returns zero.
>>> class Foo:
... def __int__(self):
... return 0
...
# create instance and covert to integer
>>> int(Foo())
0
This is a trivial example of how these magic methods word. As PEP08 mentions, you shouldn't need magic methods beyond those already defined and if you don't need special behaviors in your class definitions, you might be able to rely on the magic methods that have already been defined, like, ... "magic"!
As you'll learn later, you may wish to override a magic method, by defining a method with the same name as the magic method that was inherited from a parent class. This is not the same as creating new magic methods (those not in the official documents)>
Post back if you need more help. Good Luck!