Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial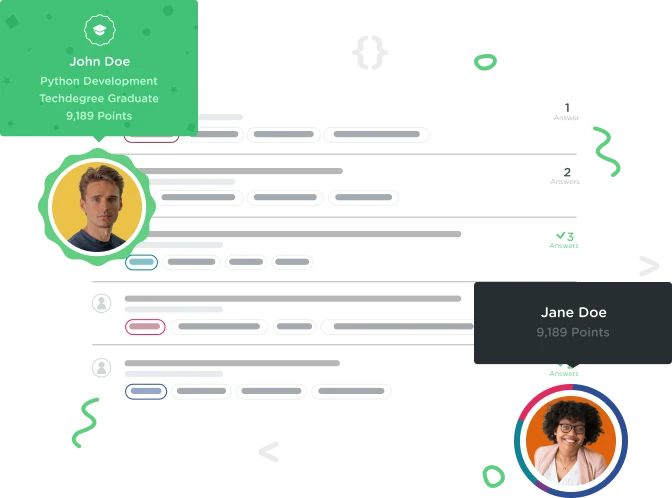

mohan Abdul
Courses Plus Student 1,453 PointsO.O.P python OK, now let's add a method named run_lap. It'll take a length argument. It should reduce the fuel_remaining
something isn't really clicking together heres second try (Steven Parker, could you please help or any one else please?) :
class RaceCar:
def __init__(self, color, fuel_remaining, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(self, length, fuel_remaining, total_length, total):
count = 0
self.laps = self.run_lap
count = +1
self.length = self.length
self.total_length = self.length * 0.125
self.total = self.length - self.fuel_remaining
class RaceCar:
def __init__(self, color, fuel_remaining, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(self, length):
count = 0
self.laps = run_lap
count = +1
self.length = 0.125
self.length - self.fuel_remaining
1 Answer
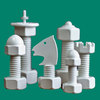
Steven Parker
231,269 PointsHere's a few hints:
- you won't need "count" ("laps" does that job)
- but you should create "laps" with the other instance variables, and set it to 0 there
- "length" is a parameter, not an instance variable (so no "self.length")
- you won't modify "length" , but you will use it to modify "fuel_remaining"
- make sure the fuel formula matches the instructions
mohan Abdul
Courses Plus Student 1,453 Pointsmohan Abdul
Courses Plus Student 1,453 Pointsthis is what i got so far, with self.total_length and self.total_fuelremaining i get this error message, "AttributeError: 'RaceCar' object has no attribute 'total_length'." even if i take away the instance variable self.
mohan Abdul
Courses Plus Student 1,453 Pointsmohan Abdul
Courses Plus Student 1,453 Pointswhy doesn't length need an instance variable. in the initfunction method color and fuel_remaining all got given an instance variable.
Steven Parker
231,269 PointsSteven Parker
231,269 PointsA few more hints:
__init__
" method with the other instance variablesmohan Abdul
Courses Plus Student 1,453 Pointsmohan Abdul
Courses Plus Student 1,453 PointsThe last two lines don't do anything.
Steven Parker
231,269 PointsSteven Parker
231,269 PointsYou're right, just performing a calculation doesn't do anything lasting. For that, you need to perform the calculation in an assignment statement that puts the result into a variable (perhaps back into one being used in the calculation).
mohan Abdul
Courses Plus Student 1,453 Pointsmohan Abdul
Courses Plus Student 1,453 Pointsi got the last two lines to do something but i still get a bummer message "Bummer: Did you adjust the
fuel_remaining
attribute?"Steven Parker
231,269 PointsSteven Parker
231,269 PointsSure enough, this code makes no changes to "fuel_remaining" but creates and returns "total_fuel" instead. But that's not what the instructions ask for. So to conform to the instructions:
mohan Abdul
Courses Plus Student 1,453 Pointsmohan Abdul
Courses Plus Student 1,453 PointsWith out return nothing happens, can you show me how to do it with out return? I have no idea how create a count for self.lap do i use the enumerate or f' string index? . I neatened the formula so only length is used once but i have encountered another problem, if enter the following in the console it only takes 1. 25 away: >>> mohan=RaceCar("blue", 56)
Steven Parker
231,269 PointsSteven Parker
231,269 PointsThe fuel calculation seems correct now. I see how you are using "return" for testing, but it's not needed for the challenge.
The code for a typical method to increment the laps would look like this:
self.laps += 1
mohan Abdul
Courses Plus Student 1,453 Pointsmohan Abdul
Courses Plus Student 1,453 Points'''self.laps=0''' has to be set to zero and increment by one from there, so does it look like this: (it comes back with "Bummer: Be sure to set the
laps
attribute to 0")Steven Parker
231,269 PointsSteven Parker
231,269 PointsSetting it to 0 is done in the "
__init___
" method. But the increment should be done only in the "run_lap
" method.mohan Abdul
Courses Plus Student 1,453 Pointsmohan Abdul
Courses Plus Student 1,453 PointsI tried to put it through and it comes back with, "Bummer: Hmm, some attributes didn't get set correctly".
Steven Parker
231,269 PointsSteven Parker
231,269 PointsYour original code had some lines in the "
__init__
" method that set additional keyword arguments using "setattr". But apparently during the other changes, that bit of the code got removed.Put it back in and you should pass the challenge.