Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial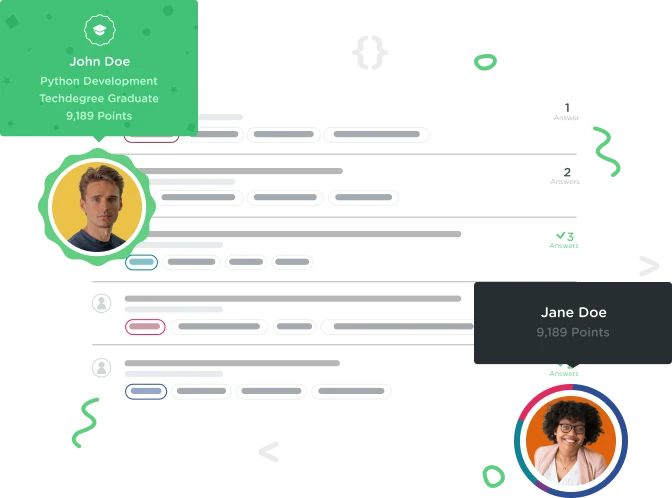

bedriye kurt
902 PointsOOP Quiz - Cant Figure Out The problem
Here is my code, everything looks fine, ı can see the problem, any one to help?
<?php
class Fish
{
public $common_name;
public $flavor;
public $record_weight;
public function getInfo()
{
echo "A" . $this->common_name . "is an" . $this->flavor . "ed fish. The world record weight is " . $this->record_weight;
}
}
$bass = new Fish();
$bass->common_name = "Largemouth Bass";
$bass->flavor = "Excellent";
$bass->record_weight = "22 pounds 5 ounces";
$bass->getInfo();
?>
5 Answers
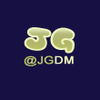
Jonathan Grieve
Treehouse Moderator 91,253 PointsIt looks like you need to feed your variables into the code first before you try to output their results.
<?php
$bass = new Fish();
$bass->common_name = "Largemouth Bass";
$bass->flavor = "Excellent";
$bass->record_weight = "22 pounds 5 ounces";
$bass->getInfo();
class Fish
{
public $common_name;
public $flavor;
public $record_weight;
public function getInfo()
{
echo "A" . $this->common_name . "is an" . $this->flavor . "ed fish. The world record weight is " . $this->record_weight;
}
}
?>
Now that the variables have been assigned first in this example the getinfo(); has values to echo the code to the browser, since the code compiles top to bottom. :-)

bedriye kurt
902 PointsAww.. Thank you man ! Appreciate the quick response :)
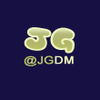
Jonathan Grieve
Treehouse Moderator 91,253 Pointsnp, glad to help :)

bedriye kurt
902 PointsBut, ı tried the code and it gives me the same error :(
err msg : Be sure you include the fish's common name in the return value from getInfo. Use $this to reference the current object.
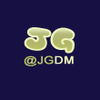
Jonathan Grieve
Treehouse Moderator 91,253 PointsHmmm bit trickier than I thought then. :/
It looks like this challenge is from a course I've not yet taken so that's what caught me out a bit. Here's a response to a similar challenge from another course that might help you. https://teamtreehouse.com/community/oop-quiz-cant-figure-out-the-problem
Declare the variables first, Add your getinfo() method and then echo out the new string as an instance of the Fish object.
<?php
class Fish {
public $common_name = '';
public $flavor = '';
public $record_weight = '';
function __construct($name, $flavor, $record){
$this->common_name = $name;
$this->flavor = $flavor;
$this->record_weight = $record;
}
public function getInfo() {
return "A " . $this->common_name . " is an " . $this->flavor .
" flavored fish. The world record weight is " . $this->record_weight . ".";
}
}
$bass = new Fish('Largemouth Bass', 'Excellent', '22 pounds 5 ounces');
echo $bass->getInfo();
?>
Good luck.

bedriye kurt
902 PointsWorked like charm , thanks buddy :)