Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial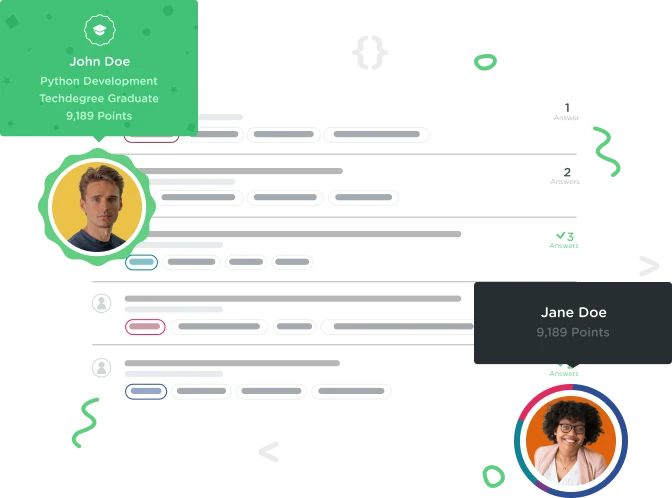

Adam Del Conte
7,250 PointsOOP RPG-dice YatzyScore: So proud of working out many issues on my code! Learned so much BUT MATH STILL WRONG? :(
Hello all!
Contrary to what many older posts have said, this final challenge has really helped me solidify a lot of the concepts here. I know I have a lot left to learn, and a lot of room for deeper understanding about the usage of these classes, decoraters, the super() function, etc. But overall I am really proud of the work I've done in creating a program that runs. It took several times watching through and working through the code line by line, but I identified and corrected several typos, indent errors, and even a few Attribute and ValueErrors :) For someone who hadn't written a line of code since C++ in my highschool CS class 20 years ago, this feels huge.
HOWEVER, when I run the final example, I get 12 instead of 8.
Maybe I'll see the answer when I look at it after a nights sleep, but I am stumped at the moment.
Any insight into why it is returning this score would be welcome.
Here is what I'm putting in the console:
from hands import YatzyHand
from dice import D6
from scoresheets import YatzyScoresheet
hand = YatzyHand()
three = D6(value=3)
four = D6(value=4)
one = D6(value=1)
hand[:] = [one, three, three, four, four]
YatzyScoresheet().score_one_pair(hand)
12
And here is the rest of the code:
dice.py
import random
class Die:
def __init__(self, sides=2, value=0):
if not sides >= 2:
raise ValueError('Must have at least 2 sides')
if not isinstance(sides, int):
raise ValueError('Sides must be a whole number')
self.value = value or random.randint(1, sides)
def __int__(self):
return self.value
def __eq__(self, other):
return int(self) == other
def __ne__ (self, other):
return int(self) != other
def __gt__ (self, other):
return int(self) > other
def __lt__(self, other):
return int(self) < other
def __ge__(self, other):
return int(self) > other or int(self) == other
def __le__(self, other):
return int(self) < other or int(self) == other
def __add__(self, other):
return int(self) + other
def __radd__(self, other):
return int(self) + other
def __repr__(self):
return str(self.value)
class D6(Die):
def __init__(self, value=0):
super().__init__(sides=6, value=value)
hands.py
from dice import D6
class Hand(list):
def __init__(self, size = 0, die_class=None, *args, **kwargs):
if not die_class:
raise ValueError('You must provide a die class')
super().__init__()
for _ in range(size):
self.append(die_class())
self.sort()
def _by_value(self, value):
dice = []
for die in self:
if die == value:
dice.append(die)
return dice
class YatzyHand(Hand):
def __init__(self, *args, **kwargs):
super().__init__(size=5, die_class=D6, *args, **kwargs)
@property
def ones(self):
return self._by_value(1)
@property
def twos(self):
return self._by_value(2)
@property
def threes(self):
return self._by_value(3)
@property
def fours(self):
return self._by_value(4)
@property
def fives(self):
return self._by_value(5)
@property
def sixes(self):
return self._by_value(6)
@property
def _sets(self):
return {
1: len(self.ones),
2: len(self.twos),
3: len(self.threes),
4: len(self.fours),
5: len(self.fives),
6: len(self.sixes)
}
scoresheets.py
class YatzyScoresheet:
def score_ones(self, hand):
return sum(hand.ones)
def score_twos(self, hand):
return sum(hand.twos)
def score_threes(self, hand):
return sum(hand.threes)
def score_fours(self, hand):
return sum(hand.fours)
def score_fives(self, hand):
return sum(hand.fives)
def score_sixes(self, hand):
return sum(hand.sixes)
def _score_set(self, hand, set_size):
scores = [0]
for worth, count in hand._sets.items():
scores.append(worth*set_size)
return max(scores)
def score_one_pair(self, hand):
return self._score_set(hand, 2)
Thanks in advance! Cheers!
1 Answer
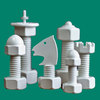
Steven Parker
229,732 PointsIn the last line of scoresheets.py:
return self._score_set(hand, 2)
Note that _score_set is always being called with a set_size of 2, and since 2 sixes is the largest value of any pair, the result is 12. Clearly, you'll want to evaluate the actual number of dice of a particular value that exist in the hand.
And for future questions, when sharing large or multiple files it's much better to make a snapshot of your workspace and post the link to it here. That makes it far easier to replicate your issue.
Adam Del Conte
7,250 PointsAdam Del Conte
7,250 PointsThank you Steven! Appreciate your help.
Will take note of snapshots moving forward too