Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial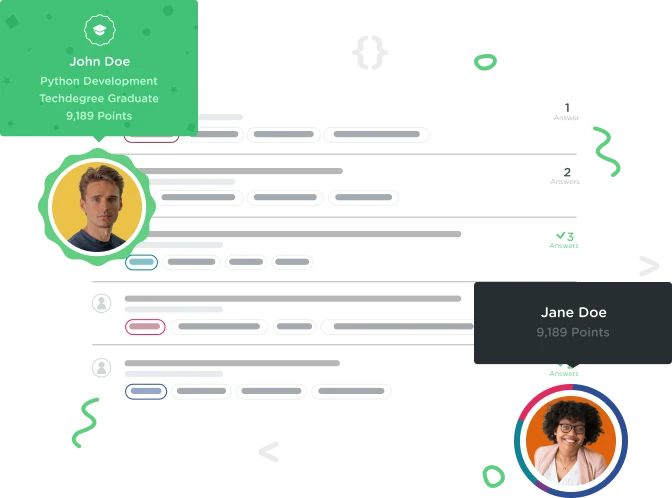

Alex Hipolito
Full Stack JavaScript Techdegree Graduate 17,582 PointsOperations on Arrays for JavaScript
Hey everyone, I found there wasn't an outline of Operations on Arrays for JavaScript. I thought I'd add my notes here in case anyone found it useful. You can try this out using the browser console in Chrome DevTools.
These notes are not comprehensive, please refer to the excellent JS courses on Treehouse and the MDN documentation for more information. Happy to take in any feedback as well.
JavaScript
Accessing Elements
Similar to Python, use the index operator on a list to access values using an index. Note that index values start at 0.
let numbers = [1, 2, 3, 4, 5, 6];
numbers[2]
// returns 3
Using an index that is out of bounds of the current range of values returns undefined
.
numbers[10];
// returns undefined
List Length
To determine the number of items in a list, use the Array.length
property:
numbers.length
// returns 6
To iterate of each item in the list:
for (let i = 0; i < numbers.length; i++) {
console.log(numbers[i]);
}
/* returns
1
2
3
4
5
6
*/
Array Operations
To append to the array, use the Array.push()
method:
numbers.push(7)
numbers
// returns (7) [1, 2, 3, 4, 5, 6, 7]
To concantenate one array with another, use Array.concat()
method:
numbers = numbers.concat([8, 9, 10]);
// returns (10) [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
To insert an element to the array, use Array.splice()
:
numbers.splice(10, 0, 11)
numbers
// returns (11) [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11]
To remove the last item from the array, use Array.pop()
method:
numbers.pop()
numbers
// returns (10) [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Or if you want to remove an item within the array, you can also use Array.splice()
method for this purpose:
numbers.splice(2, 1)
numbers
// returns (9) [1, 2, 4, 5, 6, 7, 8, 9, 10]
Array Membership
To test for membership in a list, use Array.findIndex()
method:
numbers.findIndex((number) => number === 5);
// returns 3
numbers.findIndex((number) => number === 30);
// returns -1
Arrays in JavaScript are also mutable, which means any element can be updated using the index operator along with index position.
numbers[0] = 22
numbers
// returns (9) [22, 2, 4, 5, 6, 7, 8, 9, 10]
Hope you find these useful!
1 Answer
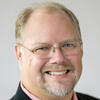
Jason Larson
8,361 PointsDon't forget about shift() and unshift() to remove or add elements to the beginning of an array.
let numbers = [1, 2, 3, 4, 5, 6];
numbers.shift()
numbers
// returns (5) [2, 3, 4, 5, 6]
numbers.unshift(1)
numbers
// returns (6) [1, 2, 3, 4, 5, 6]
There are also some nice new methods for arrays, like .filter()
, .map()
, .reduce()
, and .reverse()
to name a few. W3Schools has a complete list here