Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial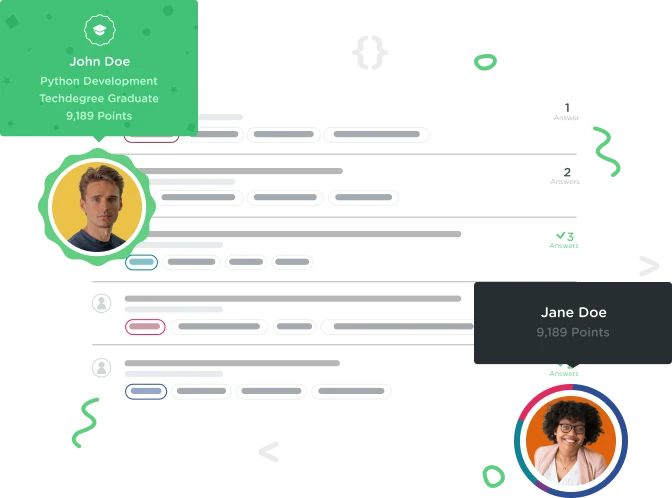
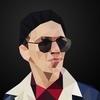
Gleb Albovsky
5,341 PointsOpposite Number Game problem
I was trying to except string and numbers > 10, but got an error:
import random
def game():
try:
secret_num = int(input("Please enter a number from 1 to 10: "))
except ValueError:
print("{} is now a number!".format(secret_num))
except secret_num > 11:
print("{} greater than 10!".format(secret_num))
guesses = []
while len(guesses) < 3:
computer_num = random.randint(1, 10)
if secret_num == computer_num:
print("Well done! The number was {}".format(secret_num))
break
else:
print(computer_num)
game()
Please say, how to except strings and numbers > 10
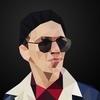
Gleb Albovsky
5,341 PointsTraceback (most recent call last):
File "opposite_number_game.py", line 8, in game
secret_num = int(input("Please enter a number from 1 to 10: "))
ValueError: invalid literal for int() with base 10: 'dfivdu'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "opposite_number_game.py", line 24, in <module>
game()
File "opposite_number_game.py", line 10, in game
print("{} is now a number!".format(secret_num))
UnboundLocalError: local variable 'secret_num' referenced before assignment
treehouse:~/workspace$
2 Answers
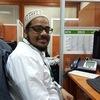
Shabbir Salumberwala
4,313 PointsOpposite Number game for reference (Try refactoring it for best practices) :
import random
import time
def game(username,Secret_Num,Guess_limit):
guesslimit = Guess_limit
while guesslimit > 0:
print("Mr. Computer. Whats your guess?")
time.sleep(3)
guess = random.randint(1,10)
if guess == Secret_Num:
print("You got it! the number was {}".format(Secret_Num))
elif Secret_Num - guess > 3:
print("Your guess {} is Too low".format(guess))
guesslimit -= 1
elif Secret_Num - guess < -3:
print ("Your guess {} is to high!".format(guess))
guesslimit -= 1
else:
print("Bummer! {} was close".format(guess))
guesslimit -= 1
if guesslimit == 1:
print("last chance Mr.Computer!\n")
time.sleep(1)
else:
print("\nThe secret number was : {}. Better Luck Next Time!".format(Secret_Num))
repeat_game = input("\n{} do you want to play again? press Y for Yes and N for No: ".format(username))
if repeat_game.lower() == "y":
main()
else:
print("bye!")
def main():
secret_Num = input("\n hey {}! Enter your secret number. Let the computer Guess :-)".format(Username))
try:
int(secret_Num)
except ValueError:
print("How do I add {} + {}?".format(secret_Num, secret_Num))
repeat_process = input("\nYou may want try again? if so, then press Y for Yes otherwise press N to end: ")
if repeat_process.lower() == "y":
main()
else:
print("bye!")
else:
if 1 <= int(secret_Num) <= 10:
game(Username,int(secret_Num),3)
else:
repeat_process = input("\n The number should be between 1-10. You may want try again? if so, then press Y for Yes otherwise press N to end: ")
if repeat_process.lower() == "y":
main()
else:
print("bye!")
Username = input("\n What is your name buddy?")
main()

Tri Pham
18,671 Points- You did greater than 11 not 10. And it only goes into an exception if there's an error. int(input("11")) is perfectly fine to do.
- If its gonna error, its gonna error with int('a non-number string') so secret_num won't get assign to anything.
You should put result = input("Please enter a number from 1 to 10: "). It should never error there. Then use result instead of secret_num in your exception
Alexander Davison
65,469 PointsAlexander Davison
65,469 PointsWhat's the error?