Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial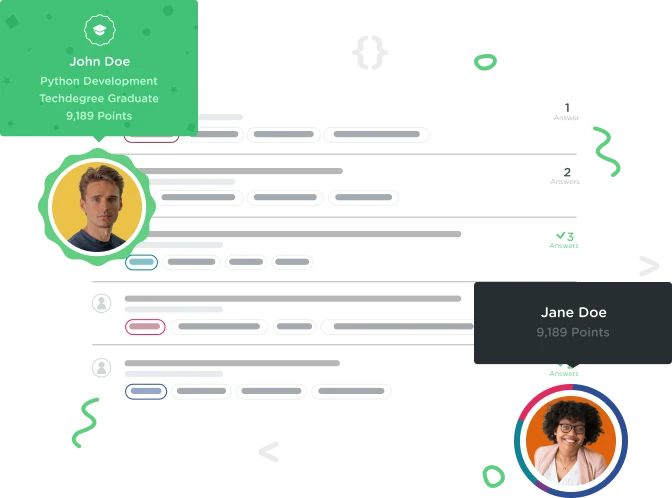

luke jones
8,915 PointsOptional Bindings Task
In the editor, you have a pretty complex dictionary representing a movie. It contains a nested dictionary, which itself contains an array of Strings as a value.
Your job is to assign the string containing the value for the lead actor (which for the sake of the example is at position 0 in the array) to the variable leadActor.
Since dictionaries return optional values, you will have to use an if let statement to unwrap each consecutive operation.
let movieDictionary = ["Spectre": ["cast": ["Daniel Craig", "Christoph Waltz", "LÊa Seydoux", "Ralph Fiennes", "Monica Bellucci", "Naomie Harris"]]]
var leadActor: String = ""
// Enter code below
if let movieCast = movieDictionary["Spectre"],
let leadActor = movieCast["cast"] {
print(leadActor[0]) }
not sure where I stand with the variable part. Feel like I'm close but missing something simple or needs a small change
2 Answers

Martin Wildfeuer
Courses Plus Student 11,071 PointsIn your code, you assign movieCast["cast"] to leadActor, you are only printing the first actor to the console. You would really want to assign the first actor to leadActor. Let me give you the working code first:
if let movie = movieDictionary["Spectre"],
let cast = movie["cast"] {
leadActor = cast[0]
}
The important difference here is that I am not using the variable name leadActor for the result of an optional unbinding. What Swift really does is creating a new variable leadActor explicitly for the scope of the if statement (the actual unbinding). This way, you can't access the top level leadActor variable you created before. Put the following example in a playground and you will see what I mean:
var test = "This won't be changed"
var strg: String? = "That's another scope indeed"
if let test = strg {
print(test) // Outputs "That's another scope indeed"
}
print(test) // Outputs "This won't be changed"
Hope that helps.

luke jones
8,915 PointsYeah that has helped a lot! I see I need to declare the variable inside the console else the string inside the variable leadActor is still empty. Makes sense.
Thanks!

Martin Wildfeuer
Courses Plus Student 11,071 PointsPerfect! Glad I could help :)