Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial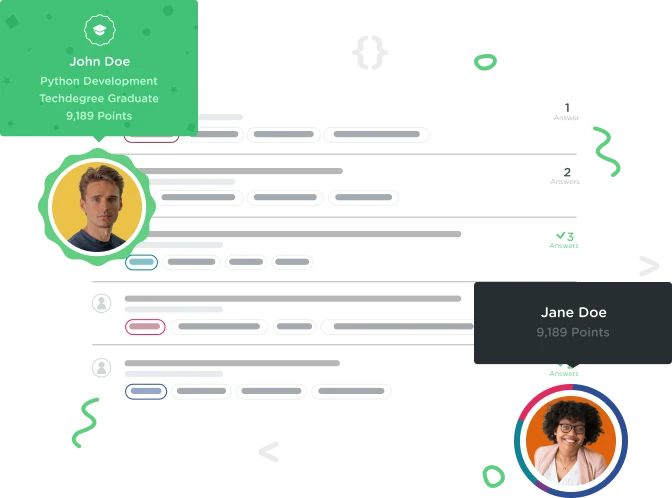

tytyty
4,974 PointsOptional parameter underscore? An underscore means that this parameter does not have an external parameter.
Amit said, "And since in this case we are not going to have any parameter, I mean the parameter is gonna be optional, we need to specify an underscore. An underscore means that this parameter does not have an external parameter".
What does he mean 'external parameter'? Does he mean %value? Where in the code would this external parameter be if there was one? Would it be a variable or constant of the Product class or the init?
class Product {
let title: String
var price: Double = 0.0
init(title: String, price: Double) {
self.title = title
self.price = price
}
func discountedPrice(percentage: Double) -> Double {
return price - (price * percentage / 100)
}
}
enum Size {
case Small, Medium, Large
init() {
self = .Small
}
}
class Clothing: Product {
var size = Size()
override func discountedPrice(_ percentage: Double = 10.0) -> Double {
return super.discountedPrice(percentage)
}
}
2 Answers

tytyty
4,974 PointsThe answer is this:
Forget about optional this optional that.
In Swift if a variable is not going to be used anywhere else in the code you actually don't need to use 'var variableName'.
So for example if you are creating a NSTimer object and its only purpose is to serve as a timer and isn't going to be passed around the program you can just do this. You don't need to write 'var timer'.
_ = NSTimer.scheduledTimerWithTimeInterval(1, target: self, selector: Selector("updateTime"), userInfo: nil, repeats: true)
Swift will give you a yellow warning when a variable isn't being used anywhere else in the code.

J.D. Sandifer
18,813 PointsHe simply means that the parameter is supplied internally - within the function itself - if one is not supplied with the method call. That's why the function's parameter also includes the assignment of 10.0
to percentage. This is the value used if one is not given with the method call.
Here's an example that could follow the example code you gave:
var someClothes = Clothing(title:"Some Clothes",price:50.0)
var price:Double
price = someClothes.discountedPrice() // Valid because percentage is optional.
price = someClothes.discountedPrice(5.0) // Also a valid method call.

Nikolai Montesclaros
11,928 PointsThanks for the clarification however I am still not clear of the error in the first place. From what I gathered from StackOverFlow, the underscore in front of the first parameter is for when you call this method you do not need to supply the label along with the actual parameter value. My issue is that in Swift the first parameter is not required to be labeled by default. So if you declare the signature as discountedPrice(Percentage: Double = 10.0) shouldn't it have worked in the first place without any issues?
tytyty
4,974 Pointstytyty
4,974 PointsSo the underscore just means the value is optional and if you don't pass a value it will default to the value assigned and you can also give it any value at any time?
Where in the code would this external parameter be if there was one? External means it would be a variable or constant of the Product class or the init method?