Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial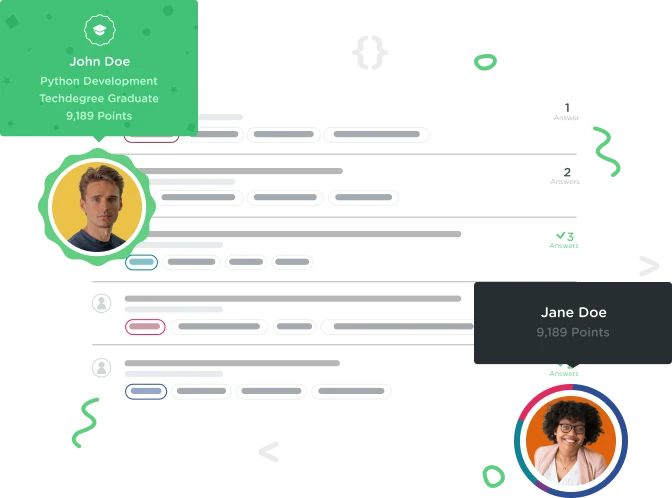
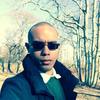
Basilio Guzmán
Courses Plus Student 1,302 PointsOptionals
Don't know what I'm doing bad here:
func search(#name: String) -> String? {
let names = ["Doc","Grumpy","Happy","Sleepy","Bashful","Sneezy","Dopey"]
for n in names {
if n == name {
return n
}
}
return nil
}
let result = ["Doc", "Grumpy", "Happy", "Sleepy", "Bashful", "Sneezy", "Dopey"]
if name = search("Doc") {
println("Found")
}
2 Answers

Chris Shaw
26,676 PointsHi Basilio,
There is a couple of issues with your code, let's going through them.
- You have a named paramater aka
#name
, whenever you have named parameters you have to refer to them in your method call - You have reassigned the array from the
search
method toresult
and haven't used a properif-let
statement
Fixing Problem 1
As I said whenever you have named parameters you need to refer to them, in the case of the search
method it's asking for the following.
search(name: "Doc")
Fixing Problem 2
First off you don't need to reassign the array to an result constant, instead you want to use an if-let
statement which checks for the optional and executes code inside the if
statement if the result isn't nil
.
if let result = search(name: "Doc") {
println("Found")
}
By the end you should have the following.
func search(#name: String) -> String? {
let names = ["Doc","Grumpy","Happy","Sleepy","Bashful","Sneezy","Dopey"]
for n in names {
if n == name {
return n
}
}
return nil
}
if let result = search(name: "Doc") {
println("Found")
}
Happy coding =)

jonathan hegranes
2,868 PointsThanks for the answer. Curious though...
What are the benefits of using a named function?
Basilio Guzmán
Courses Plus Student 1,302 PointsBasilio Guzmán
Courses Plus Student 1,302 PointsYes, I try a little more before searching this answer and I complete it. Thanks anyway for the answer.