Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial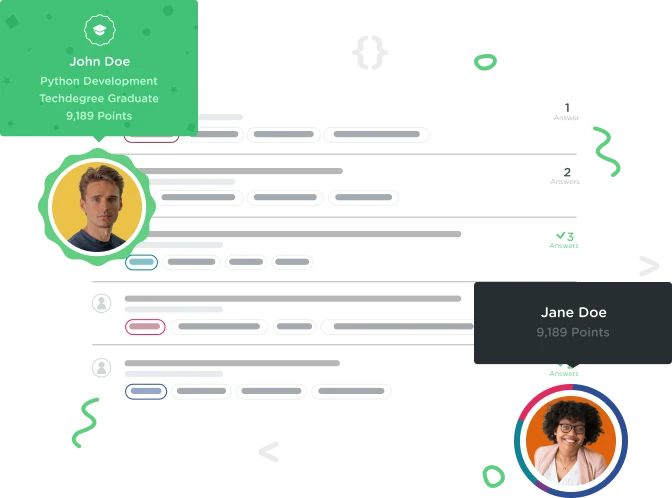
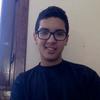
Mohammed Khalil Ait Brahim
9,539 PointsOr operator
Hi I have this question. In the video Jim does this:
name = name || "YOU";
Doesn't this expression evaluate to true or false rather than to name | "You". (In fact it would always evaluate to true)
So:
name = true;
2 Answers
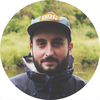
Sean Ahern
7,533 PointsHi Mohammed,
It would only qualify as true if:
- name was equal to true before that line
- You wrapped it in something that casted the value to a boolean like the following:
name = !!(name || "You");
Also, in that code sample, it would always qualify as true because the fallback value is always present as "You".
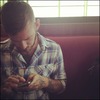
Erik McClintock
45,783 PointsMohammed,
This in itself is not a boolean statement, though it can definitely look a bit confusing with the inclusion of the OR operator. To further understand what's going on here, let's take a deeper look at the bigger picture.
What's going on at that point in the video is that Jim has created an object called 'jim' and given it a couple properties, and then he has written a method (which is the term used for functions that live within objects) called 'greet' that accepts two parameters, called 'name' and 'mood'.
If you look at the 'greet' method as any other function, you can possibly recognize a bit more easily what is going on with the parameters that he is passing in. As he states, the reason that he assigns those parameters the values of 'name = name || "You"' and 'mood = mood || "good"' is to make them optional when that 'greet' method is called. He is taking and passing those parameters (or what may be passed into the method as their arguments) in a console.log statement, and thus needs them to hold some value so the log statement makes sense. By setting them up in this manner, he is effectively giving them default values as a fallback, should they not have explicit values passed in when the method is called. It's sort of shorthand for writing out an IF conditional within the method. The same effect could be achieved by writing it out like this:
//previous code...
greet: function(name, mood) {
//if name does not equal null, i.e. if it has a value passed in, then...
if(name != null) {
//...assign the value of the name variable to the value that was passed into the method when it was called
name = name;
} else {
//...otherwise, assign the name variable a default, fallback value of "You"
name = "You";
}
//create another IF statement for checking the value of the mood argument
//rest of function here to print out the results to the console
}
You can write it in shorthand, though, by simply assigning the variable one thing OR another, depending on whether that first thing is present at all.
It may help to illustrate this if you open up your development console in your browser, paste in the following code, and then try adding/removing arguments in the call on the 'greet' method:
var person = {
greet: function(name, mood) {
if(name != null) {
name = name;
} else {
name = "You";
}
if(mood != null) {
mood = mood;
} else {
mood = "good";
}
console.log("name = " + name + ", mood = " + mood);
}
};
//calling the 'greet' method without any arguments, thus their values will go to the defaults that we set
person.greet();
//calling the 'greet' method WITH arguments, thus their values will be captured and passed into our console.log statement
person.greet('erik', 'happy');
Copy and paste that into your console, and you should see two different statements printed: one with the default answers, and one with the arguments we passed in. Now, here is that same code, but with the IF statements rewritten to assign values to those parameters in the manner that Jim did (copy and paste this in, and you'll see that you get the same results as above):
var person = {
greet: function(name, mood) {
name = name || "You";
mood = mood || "good";
console.log("name = " + name + ", mood = " + mood);
}
};
//calling the 'greet' method without any arguments, thus their values will go to the defaults that we set
person.greet();
//calling the 'greet' method WITH arguments, thus their values will be captured and passed into our console.log statement
person.greet('erik', 'happy');
It can definitely be a bit confusing at first, since there are so many ways to write the same thing, but hopefully this helps to clarify things a little bit.
Erik
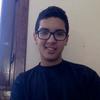
Mohammed Khalil Ait Brahim
9,539 PointsHi Eric Thank you for your answer I did get the purpose of what Jim was doing but thing is I wasn't not sure whether that statement would assign to name the string rather than the true value. Now I understand how it works
Mohammed Khalil Ait Brahim
9,539 PointsMohammed Khalil Ait Brahim
9,539 PointsYes I know 'cause "You" is a true value but I just had some doubts it's clear now that if you don't explicitly cast the it assigns the first true value in the expression to name right ?
Sean Ahern
7,533 PointsSean Ahern
7,533 PointsThat's correct. Here's some more detailed information about how boolean operators behave with non-boolean comparisons.
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Logical_Operators