Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial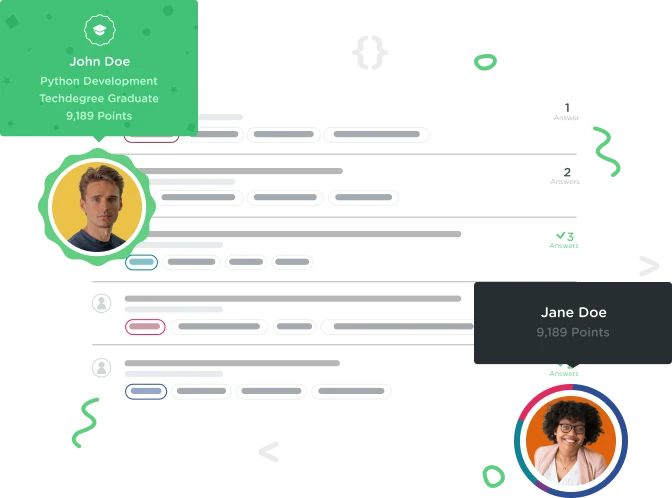

Greg Schudel
4,090 Pointsorder matters in javascript function?
I'm on the object orient programming course with javascript. I still consider myself a beginner.
I'm learning things about order. Here is my snap shot, but my question is really specific. https://w.trhou.se/g7a81vktok
In my playlist.js file, between lines 24 to 34 I previously had this:
Playlist.prototype.next = function() {
this.stop();
this.nowPlayingIndex++;
this.play();
if(this.nowPlayingIndex == this.songs.length) {
this.nowPlayingIndex = 0; // why are we turning this to zero, shouldn't we tell it to just stop?
}
};
I would get these errors in the console, something like this: 'play undefined' and the player wouldn't alternate between the songs and movies all the way (it would only hight the second and third entry ).
but re-watched the video and changed it to this
Playlist.prototype.next = function() {
this.stop();
this.nowPlayingIndex++;
if(this.nowPlayingIndex == this.songs.length) {
this.nowPlayingIndex = 0; // why are we turning this to zero, shouldn't we tell it to just stop?
}
this.play(); //moved this below the about conditional
};
Now the error vanished and the player works fine, even if I put the code back. Any reason why?
2 Answers

jared eiseman
29,023 PointsWhat Gregory said. To put it another way:
Let's suppose we have an array of length 5: var myArray = [1,2,3,4,5]; myArray.length would evaluate to 5. However, the last index in the array is 4, as javascript arrays start at 0.
The check of if (myArray.length === currentIndex) checks to see if the index is now outside the actual bounds of the array, and if it is, move it back to the beginning to start the "loop" over again. Attempting to play before hand would result in it attempting to "play" an undefined var.

Greg Schudel
4,090 PointsI see. So that code..
if(this.nowPlayingIndex == this.songs.length) {
this.nowPlayingIndex = 0; // why are we turning this to zero, shouldn't we tell it to just stop?
}
Gives the player the ability to cycle around from the GUI correct?
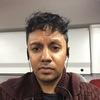
Akash Sharma
Full Stack JavaScript Techdegree Student 14,147 PointsYour
this.play()
line will try to assign an undefined value (Array out of bounds error) and thus you are dealing with undefined object.
The line javascript this.nowPlayingIndex++
just increases by one and then you check it against the songs length which is an integer (+1 of the last index value) and if that is true there is no next in your array so just set it back to the first index (Song).
GREGORY ASSASIE
3,898 PointsGREGORY ASSASIE
3,898 PointsHello, Greg. The order or the lexical environment of a function(where a function sits in your code) matter depending on whether the function body is a statement or an expression. In your case, the order shouldn't matter. however
this is slightly problematic. thus you incremented the index and played right after. should your array length = 5.
this.nowPlayingIndex++;
could reach 6 or more without a check. and this.song[6] may be undefined. hence the check to reset the counter to 0 is a necessity before you play any video
I hope this is clear.