Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial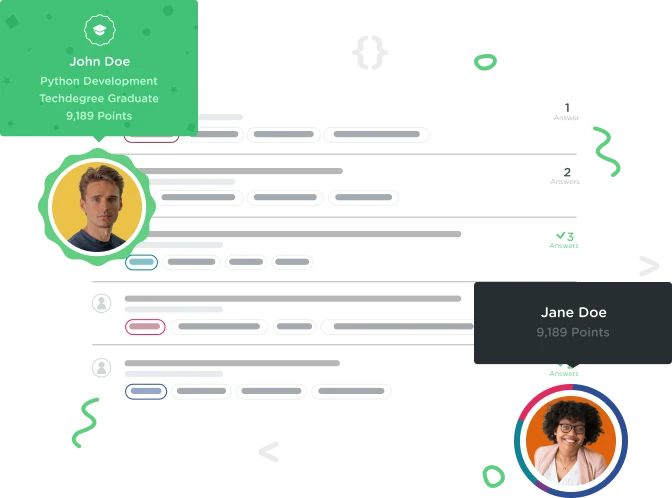

Hayden Marcus
9,181 PointsOrder of unshift.
I am wondering why the unshift method and the push method don't add multiple entries in the opposite order. In the video for Adding Data to Arrays the example pushed 'Respect' and 'Imagine' to the end of the list array and unshifted 'Louie Louie' and 'Maybellene' to the front. For the push method they were added in order, 'Respect' first and then 'Imagine', which makes sense if the code runs in order. But the unshift method also added it's items in order, which confuses me because if the code runs in order than it should add it's first argument to the front of the list array and then the second argument would be added in front again so that the order of arguments would be reversed upon output. It doesn't do this though and I'm wondering why.
2 Answers
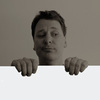
Sean T. Unwin
28,690 PointsThink of the contents between the brackets as a package -- it is a single item or container, but there can be multiple other items within that container.
Each time unshift()
is called, whatever is inside the brackets is a package containing one or many items to be added to the beginning of another package - the existing Array. The order remains the same as it was given.
Another way to think of it is as a series of trains, with a train being a package of rail cars, entering a tunnel and then emerging as a single train. Each time unshift()
is called it's adds a different train to the front of an existing one.
- Let's say we have two trains, one at each end of a tunnel.
- The trains are facing the same direction -- the engines both point West.
- The train on the East side enters first. This is the primary train that others will attach to.
- The train on the West enters next by backing into the tunnel (they are facing the same direction, remember).
- Inside the tunnel the two trains connect -- the West train's caboose connects to the East train's engine.
- When they emerge from the tunnel out from the West side, the cars of the second train (the one which entered in reverse) exits first, but it doesn't come out caboose first -- the order is the same as it was when it entered.
- Since both trains connected inside the tunnel and have now exited, we don't have two trains anymore. Now we have one long train.
So when Dave McFarland uses playlist.unshift('Louie Louie', 'Mabellene');
those two items are part of a single package (a list of one or more songs) being passed to the playlist
Array. In other words, unshift()
is taking a list of songs and adding them, in the same order given, to the beginning of the playlist to make a longer list.
This means that an Array could be considered a West-facing train and unshift()
is a way to connect one train to another (whether that new train has one car, an engine I suppose, or many cars) in such a way that the latest train connected is now the front of the existing, but now longer train.
If there are comma-separated items within the brackets of unshift()
, then this is essentially a train with multiple cars which is to be connected to an existing train to make one longer train.
The same is true for push()
except we are adding cars to the East side (or the end) of the existing train. In each situation the trains that are being combined remain in the same order as they were before connecting.

Iain Diamond
29,379 PointsPerhaps a couple of examples might help.
If you're dealing with requests, say, in the order they arrived you would want to add new items to the end of the list but process from the start, which is referred to as First In First Out (FIFO). Whereas if you were dealing with a stack based system (thinking of the good ol' stack of plates analogy), you'd want to process the last thing you pushed on to the list first, or Last In First Out (LIFO).
So having functions that can operate on either end of a list are very useful:
- push() adds to the end of an array
- unshift() adds to the start of an array
> var list = [];
undefined
> list
[]
> list.push('a');
1
> list
[ 'a' ]
> list.push('b');
2
> list
[ 'a', 'b' ]
> list.unshift('c');
3
> list
[ 'c', 'a', 'b' ]
> list.unshift('d');
4
> list
[ 'd', 'c', 'a', 'b' ]
>

Hayden Marcus
9,181 PointsI'm not sure this is addressing my question. I'm interested in why the unshift method adds things 'backwards' if called one at a time, as in your example, and 'forwards' if called with multiple arguments at once. The 'c' and 'd' in your example would be reversed if they were put into the same call to unshift.
Thanks for the info anyway.
Hayden Marcus
9,181 PointsHayden Marcus
9,181 PointsGreat answer, thanks!