Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial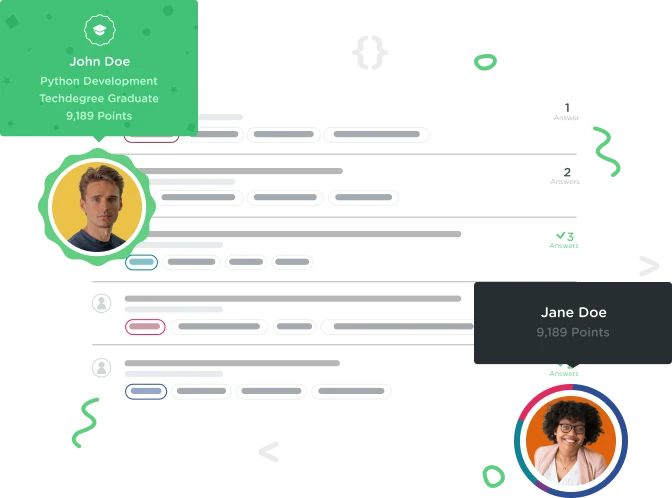
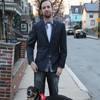
Nick Lenzi
7,979 Pointsos.system('cls') messing with my loop? Loop is fine without the clear screen command. Can someone explain?
So I started making a Deck of cards generator and then expanded it to a go fish game. Most of it is finished(still trying to work out how to have multiple references to one card name ie. jack of spades = j) but I've found this bug explained in the title and if I comment the line out the loops are fine. What's the deal? I know I could cheat by just using a print('n\ * 100') but I'd rather learn why this isn't working the way it should.
Also, any tips or suggestions to simplify my code or avoid future issues feel free to comment. I love learning all the pitfalls to avoid and how they work internally. Thanks!
import random
import os # os CLS function messing with loops
card_names = 'two,three,four,five,six,seven,eight,nine,ten,jack,queen,king,ace'
card_names = card_names.split(",")
card_range = ['2','3','4','5','6','7','8','9','10','J','Q','K','A']
hearts = [card + ' of Hearts' for card in card_range]
spades = [card + ' of Spades' for card in card_range]
clubs = [card + ' of Clubs' for card in card_range]
diamonds = [card + ' of Diamonds' for card in card_range]
# find utf-8 code for corresponding symbols for display.
deck = hearts + spades + clubs + diamonds
player_hand = []
opponent_hand = []
opponent_guess_pool = []
def player_draw(): # working
drawn_card = random.choice(deck)
player_hand.append(drawn_card)
deck.remove(drawn_card)
def opponent_draw(): # working
drawn_card = random.choice(deck)
opponent_hand.append(drawn_card)
deck.remove(drawn_card)
def new_hand(): # working
for player_hand in range(0, 5):
player_draw()
for opponent_hand in range(0, 5):
opponent_draw()
def game():
new_hand()
player_points = 0
opponent_points = 0
while True:
while True:
if len(deck) != 0:
#os.system('cls') # Problem with clear screen command
print("Drawing card...")
player_draw()
print("Your hand: \n"); print(player_hand)
print(opponent_hand) # TEMP----------------------->
player_guess = input('Guess a card: > ')
print(player_guess) # TEMP-------------------------->
if player_guess in opponent_hand:
player_points += 1
print(player_points) # TEMP----------------------->
opponent_hand.remove(player_guess)
player_draw
elif player_guess not in opponent_hand:
if player_guess not in deck:# or player_guess not in card_names:
print ("That's not a card I've ever heard of... Guess again!")
else:
print("Go fish!")
player_draw()
print("Your hand: \n"); print(player_hand)
break
else:
game_over()
while True:
if len(deck) != 0:
opponent_draw()
opponent_guess_pool = player_hand + deck
opponent_guess = random.choice(opponent_guess_pool)
print("Hmmmm I pick... {}!".format(opponent_guess))
if opponent_guess in player_hand:
opponent_points += 1
player_hand.remove(opponent_guess)
opponent_draw()
else:
print("No??? awwwwww lame. Guess I have to go fish.")
opponent_draw()
break
else:
game_over()
def game_over():
while True:
if player_points > opponent_points:
print("You win!")
elif opponent_points > player_points:
print("Beter luck next time! You lose.")
else:
print("We tied! No way! I guess we'd better play again!")
new_game = input("Do you want to play again?(Y/n) >").lower
if new_game == "y":
game()
elif new_game == "n":
quit()
else:
print("You must enter Y or N")
game()
2 Answers
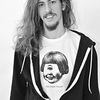
eck
43,038 PointsMight be that your are attempting to use the incorrect method for your OS. If your are running your script on a windows machine then os.system('cls')
should work, but if you run it on Linux or Mac then you need to call os.system('clear)
.
I believe that you can include a conditional in your script that checks the OS so you can call cls
the right way. This is true if the system is Windows: if os.name == 'nt'
.
if os.name == 'nt':
os.system('cls')
else:
os.system('clear')
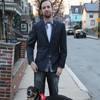
Nick Lenzi
7,979 PointsYeah luckily I do understand that bit. I left it out because I am using windows and wasn't planning on it running on other computers considering I am only doing as a learning exercise. I also am running it in powershell if that makes any difference which seems to use "clear" instead of "cls" althought trying that in the code and running it just causes an error saying it doesn't recognize the name.
The funny thing is that it is technically clearing the screen each time but it doesn't allow the loop to continue though properly. It's as if it's resetting to the beginning of the loop early.
eck
43,038 Pointseck
43,038 PointsYou could also use a ternary express to be a bit more concise:
os.system('cls' if os.name == 'nt' else 'clear')
.