Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial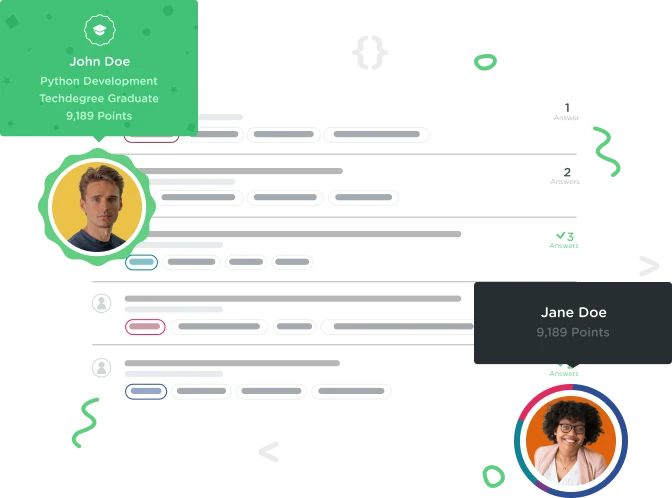

Ben Colgan
22,695 Pointsother classes not seeing xml elements in android/java
Ive just built my first android app a simple counting app that flashes the background colour green when counting up, red when going down and black if the count is at zero and stops the counter going below zero, everything is working fine the problem I'm having is there's a lot of reused code when it comes to managing the background colour change but if I try to refactor the code to make a method in a separate class to handle the background colours it can't see the background in the main activity and I can't even make the object using the id the second class just can't seem to see anything from the xml or main activity class and so I can't seem to adjust the background colour outside the main activity class and with an hour or so looking around for possible solutions I'm a little lost at how I'm supposed to get it to work.
This is the working code below before trying to refactor.
''' public class MainActivity extends AppCompatActivity {
// Initialisers
public ConstraintLayout background;
private TextView counter;
private Button plus;
private Button minus;
final Handler handler = new Handler();
int currentCount;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// ID links
counter = findViewById(R.id.count);
plus = findViewById(R.id.plusButton);
minus = findViewById(R.id.minusButton);
background = findViewById(R.id.layout);
// --Plus button--
// Increases the count by one per click
plus.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
currentCount++;
counter.setText(currentCount + "");
// Flashes the background green when the button is clicked
background.setBackgroundColor(Color.parseColor("#51b46d"));
handler.postDelayed(new Runnable() {
@Override
public void run() {
background.setBackgroundColor(Color.WHITE);
}
}, 200);
}
});
// --Minus button--
// Decreases the count by one but stops the count going below 0
minus.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
if(currentCount > 0) {
currentCount--;
counter.setText(currentCount + "");
// Flashes the background red when the button is clicked
background.setBackgroundColor(Color.parseColor("#e15258"));
handler.postDelayed(new Runnable() {
@Override
public void run() {
background.setBackgroundColor(Color.WHITE);
}
}, 200);
}else{
// If the counter is zero
background.setBackgroundColor(Color.parseColor("#000000"));
counter.setTextColor(Color.parseColor("#ffffff"));
handler.postDelayed(new Runnable() {
@Override
public void run() {
background.setBackgroundColor(Color.WHITE);
counter.setTextColor(Color.parseColor("#000000"));
}
}, 200);
}
}
});
}
} '''
1 Answer
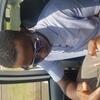
Malcom T.L Musuma
11,363 PointsHello, i'm not sure how this works but an extra tip is to confirm that you create a new class not a new activity because that way the resources will be referring to the newactivity.xml insteade of activity_main.xml