Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial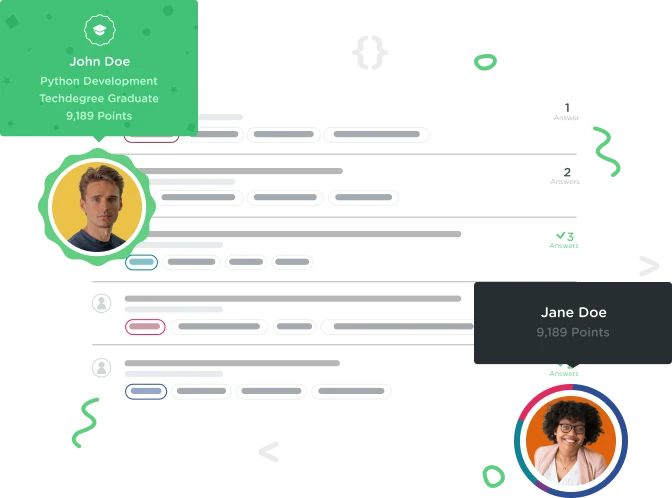
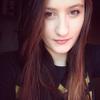
Jennifer Gerbl
8,772 PointsOther way of declaring onClickListener
On research I saw this way of declaring the OnClickListener:
showFactButton.setOnClickListener( new Button.OnClickListener(){
public void onClick(View view){
} //end onClick
}); //end setOnClickListener
So what's the difference? And what is more common in programming?
1 Answer
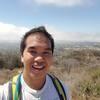
Ben Junya
12,365 PointsThis is a really great question.
In Android, there's 1000+ ways to do the exact same thing, with different pros and cons to each way.
What you did here is the most common way of making an OnClickListener for an individual button:
showFactButton.setOnClickListener(new View.OnClickListener {
@Override
public void onClick(View v) {
// Do something on click
} //end onClick
} //end new OnClick
Let's say you have a bunch of buttons that all do the same thing - Instead of writing 3 OnClickListeners, you can write just one like this:
public class MainActivity extends ActionBarActivity implements View.OnClickListener {
// Button declarations omitted for brevity
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// Button findViewById omitted for brevity
button1.setOnClickListener(this);
button2.setOnClickListener(this);
button3.setOnClickListener(this);
}
@Override
public void onClick(View v) {
// Do something when button 1, 2, or 3 is clicked
// Optionally, you can get which button was clicked by accessing the v argument that's passed to onClick
int viewID = v.getId();
}
I certainly hope this helps you out!
Ben Junya
12,365 PointsBen Junya
12,365 PointsIf you want to know a little bit of what's happening behind the scenes, an OnClickListener is what's called an interface - which acts like a callback, a common programming pattern that you'll see virtually everywhere.
It starts with this:
protected class MainActivity extends Activity implements View.OnClickListener {}
"Implements" is the keyword we're looking for here.
If you declare a new View.OnClickListener like in the first example to my answer, that means you're creating a new instance of an OnClickListener right off the bat. If you have your activity "Implement" that interface, like in the second example, you just need to pass in "this" as a parameter instead of creating a new instance.
If you want to learn more about interfaces, since they're super awesome and can do lots of cool stuff, you can read up right here: https://docs.oracle.com/javase/tutorial/java/concepts/interface.html