Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial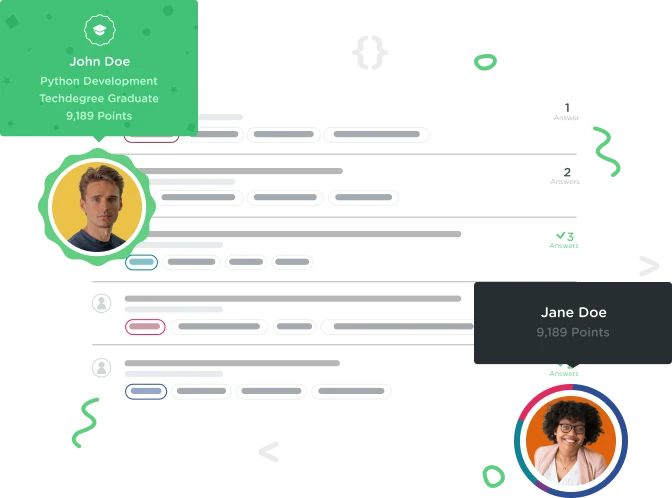
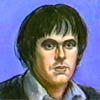
Jesse Thompson
10,684 PointsOut of curiosity what is the purpose of EventEmitter and util modules?
I dont believe we wrote this code down, I finished the project but I am wondering for usage in my own program.
It seems that usage of util.inherits is discouraged and I just cant make sense of the EventEmitter in the docs. "All EventEmitters emit the event 'newListener' when new listeners are added and 'removeListener' when existing listeners are removed." ???
Why not just parse the data normally as opposed to using this emitter?
profile.js below
var EventEmitter = require("events").EventEmitter;
var https = require("https");
var http = require("http");
var util = require("util");
/**
* An EventEmitter to get a Treehouse students profile.
* @param username
* @constructor
*/
function Profile(username) {
EventEmitter.call(this);
profileEmitter = this;
//Connect to the API URL (https://teamtreehouse.com/username.json)
var request = https.get("https://teamtreehouse.com/" + username + ".json", function(response) {
var body = "";
if (response.statusCode !== 200) {
request.abort();
//Status Code Error
profileEmitter.emit("error", new Error("There was an error getting the profile for " + username + ". (" + http.STATUS_CODES[response.statusCode] + ")"));
}
//Read the data
response.on('data', function (chunk) {
body += chunk;
profileEmitter.emit("data", chunk);
});
response.on('end', function () {
if(response.statusCode === 200) {
try {
//Parse the data
var profile = JSON.parse(body);
profileEmitter.emit("end", profile);
} catch (error) {
profileEmitter.emit("error", error);
}
}
}).on("error", function(error){
profileEmitter.emit("error", error);
});
});
}
util.inherits( Profile, EventEmitter );
module.exports = Profile;
`
1 Answer

Jeremy Ashcraft
14,786 PointsI've only started studying node since yesterday, but as far as i understand: EventEmitter is a class with many event related methods. the .emit() method lets you create a custom event. with the .emit() method you can give it a custom name, and choose what data to send along to the listener with the event when triggered. you can then set a listener for this event. the listener executes a callback function using the data passed to it via the .emit() method.
Justin Deardorff
8,448 PointsJustin Deardorff
8,448 PointsI hate to fire off documentation but you should find what you are looking for in here: https://nodejs.org/api/events.html