Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial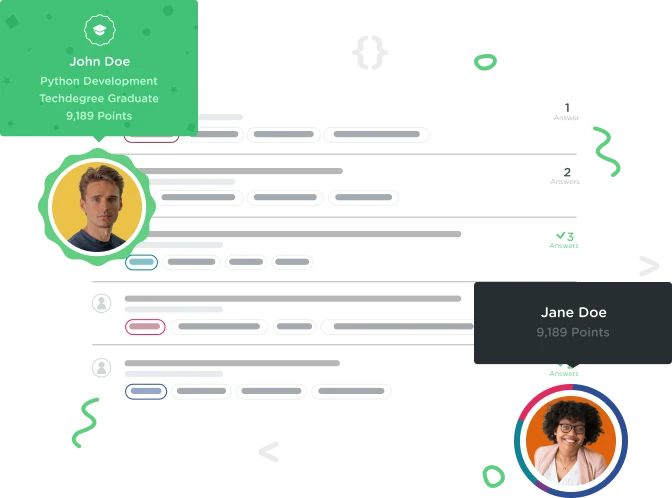

Dazznell Martinez
1,605 PointsOutcome of code not adding up correctly.
let correctAnswers = 0; let rank;
const main = document.querySelector('main');
const answer1 = prompt('Name a programming language that is also a gem?'); if (answer1.toUpperCase() === 'RUBY'){ correctAnswers += 1; } const answer2 = prompt('Name a programming language that is also a snake?'); if (answer2.toUpperCase() === 'PYTHON'){ correctAnswers += 1; } const answer3 = prompt('What programming language do you style web pages with?'); if (answer3 === 'CSS'){ correctAnswers += 1; } const answer4 = prompt('What programming language do you use to build the structure of a website?'); if (answer4 === 'HTML'){ correctAnswers += 1; } const answer5 = prompt('Which programming language can you use to build both the back end and the front end?'); if (answer5.toUpperCase === "JAVASCRIPT"){ correctAnswers += 1; }
if ( correctAnswers === 5 ) { rank = "Gold"; } else if( correctAnswers >= 3 ){ rank = "Silver"; } else if( correctAnswers >= 2 ){ rank = "Bronze"; } else { rank = "None. Please try again."; }
main.innerHTML = <h2>You got ${correctAnswers} out of 5 questions correct!<h2>
<p> Crown earned: <strong>${rank}</strong></p>
;
1 Answer
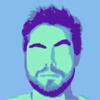
Cameron Childres
11,820 PointsHi Dazznell!
Quick tip - if you use the markdown cheatsheet linked below the comment box you can style your code to be much more readable. This makes it easier for others in the community to see your code, makes receiving assistance more likely, and prevents characters from being dropped like the `` surrounding your template literal.
Here is your code -- the only changes I've made is moving the variables to the top so I could read it a bit easier. After the code block I'll break down why it's not adding up as you expect.
let correctAnswers = 0;
let rank;
const main = document.querySelector("main");
const answer1 = prompt('Name a programming language that is also a gem?');
const answer2 = prompt('Name a programming language that is also a snake?');
const answer3 = prompt('What programming language do you style web pages with?');
const answer4 = prompt('What programming language do you use to build the structure of a website?');
const answer5 = prompt('Which programming language can you use to build both the back end and the front end?');
if (answer1.toUpperCase() === 'RUBY') {
correctAnswers += 1;
}
if (answer2.toUpperCase() === 'PYTHON') {
correctAnswers += 1;
}
if (answer3 === 'CSS') {
correctAnswers += 1;
}
if (answer4 === 'HTML') {
correctAnswers += 1;
}
if (answer5.toUpperCase === "JAVASCRIPT"){
correctAnswers += 1;
}
if ( correctAnswers === 5 ) {
rank = "Gold";
} else if ( correctAnswers >= 3 ) {
rank = "Silver";
} else if( correctAnswers >= 2 ) {
rank = "Bronze";
} else {
rank = "None. Please try again.";
}
main.innerHTML = `<h2>You got ${correctAnswers} out of 5 questions correct!<h2> <p> Crown earned: <strong>${rank}</strong></p>`;
Notice that the if statements for answer3 and answer4 do not have the .toUpperCase() method applied to them. If you want to accept "html" as an answer you'll need to convert these answers to upper case like the others. If you only wish to accept "HTML" then no change is needed.
if (answer3.toUpperCase() === 'CSS') {
correctAnswers += 1;
}
if (answer4.toUpperCase() === 'HTML') {
correctAnswers += 1;
}
Next, the if statement for answer5 includes .toUpperCase but is missing the parentheses that follow -- without them it renders the statement useless. Changing this to .toUpperCase() will allow your last answer to be properly checked.
if (answer5.toUpperCase() === "JAVASCRIPT"){
correctAnswers += 1;
}
Final note that has nothing to do with the addition: check the h2 element in your last line and make sure the closing tag includes a /
main.innerHTML = `<h2>You got ${correctAnswers} out of 5 questions correct!</h2> <p> Crown earned: <strong>${rank}</strong></p>`;
Hope this helps :)
Dazznell Martinez
1,605 PointsDazznell Martinez
1,605 PointsThanks so much, Cameron! Really appreciate it! :)