Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial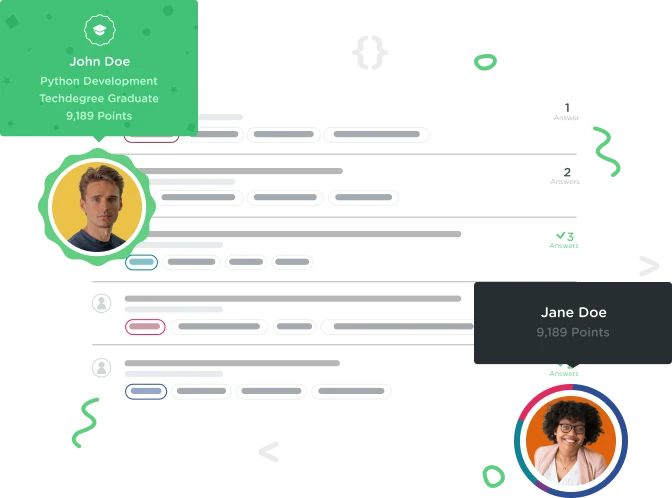

yoav green
8,611 Pointsoutput is "sleeping" even ifs false in the condition. cant understand why
class Pet {
constructor(animal, age, bread, sound){
this.animal = animal;
this.age = age;
this.bread = bread;
this.sound = sound;
}
get activity() { //getter method//
const today = new Date();
const hour = today.getHours();
if (hour > 8 && hour <=20) {
return 'playing';
} else {
return 'sleeping';
}
if (hour > 16 && hour < 23) {
return 'meeting the Bros in the hood';
} else {
return 'at home, board to death';
}
}
speak() {
console.log(this.sound);
};
}
const ernie = new Pet('dog', 1, 'pug', 'woof woof');
const vera = new Pet('cat', 5, 'persian', 'meouuu');
console.log(ernie);
4 Answers
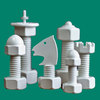
Steven Parker
230,274 PointsThe "else" action is always performed if the "if" test is false. And since both the "true" and "false" conditions cause a return, the function will end before going on to the next "if".
What you probably want is a chain of "else if" statements to check all the other possible conditions before the final "else".

yoav green
8,611 Pointsanother question. even if i erase the second "if" and "else", and the first if statement is still " if (hour > 8 && hour <=20)" i get the 'playing' return. even though it's after 20 in my time. what can be the reason?
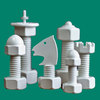
Steven Parker
230,274 PointsThat doesn't seem possible. Try this to be sure the hour is what you are expecting:
return 'playing at ' + hour;
If that doesn't reveal the issue, you can facilitate a thorough analysis if you make a snapshot of your workspace and post the link to it here.

yoav green
8,611 Pointsand i didn't manage to to preform your first answer. maybe you can help me with the code itself? do i need to create another "getter" or can i apply all the conditional statements in one "getter"? thanks alot!
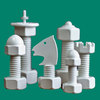
Steven Parker
230,274 PointsI assumed it would go in the same function. I added an example to my answer.

yoav green
8,611 Pointsused that - " return 'playing at ' + hour; " gave me the hour 18, even though the hour here is 21:54.
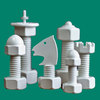
Steven Parker
230,274 PointsPerhaps the time zone is set incorrectly? You can use "new Date().getTimezoneOffset()
" to see how far off (in minutes) from UTC the system thinks you are.
yoav green
8,611 Pointsyoav green
8,611 Pointsthanks alot Steven.
Steven Parker
230,274 PointsSteven Parker
230,274 PointsHere's an example of what such a chain might look like: