Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial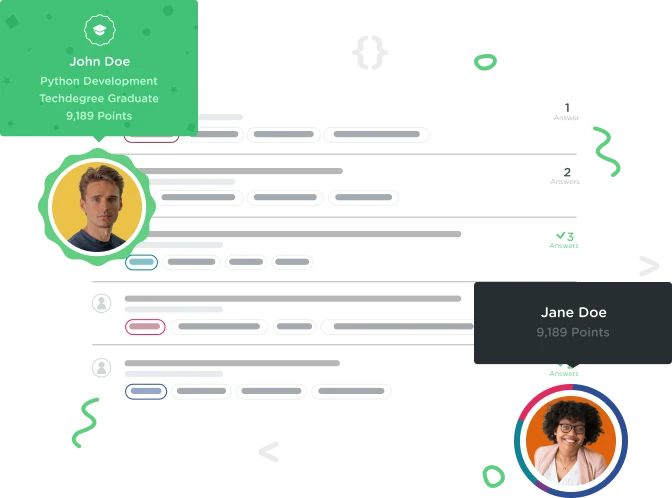

Nantheesh P
1,260 PointsOutputting python error messages
Due to the addition of the 'err method', now when the user inputs a string (such as 'two'), you not only get our personalised error message ('This is not a valid value... try again!')... but you also get a python error message ('could not convert string to float: 'two'') due to the addition of print('({})'.format(err)) in our code! How do I overcome this, yet have this code work when the user inputs int values for no. people as <=1?
Thank you,
1 Answer
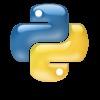
Viraj Deshaval
4,874 PointsYou can follow my below code for handling exceptions. So what this does is let's say you have two sort of exceptions in ValueError i.e.
1. >>> int("string")
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: invalid literal for int() with base 10: 'string'
2. >>> float("string")
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: could not convert string to float: 'string'
So when the string is getting converted to 'float' it throws an ValueError and when it gets converted to 'int' it throws an ValueError. This we can very well handle using try and except block. Now coming to your question, now we raised an exception ValueError after checking the below condition
if number_of_people <= 1:
raise ValueError("More than 1 person is required to split the check")
So what it did is simply we asked program to raise an exception for us and whatever the message you want to display will be handled by below block.
except ValueError as err:
This we did for our ease as we were already raising a ValueError. But it's not necessary that you should only raise ValueError, you can raise anything you want so I raised a NameError instead of ValueError and so now anyone try to pass on strings which causes ValueError will be handled by this
except ValueError:
print("Oh no! That's not a valid value. Try again...")
and if someone passes the number_of_people <= 1 then NameError gets raised and it will be handled as below:
except NameError as err:
print("({})".format(err))
See my sample code as below for your reference.
import math
def split_check(total, number_of_people):
if number_of_people <= 1:
raise NameError("More than 1 person is required to split the check")
return math.ceil(total / number_of_people)
try:
total_due = float(input("What is the total bill? "))
number_of_people = int(input("How many people are paying bill? "))
amount_due = split_check(total_due, number_of_people)
except ValueError:
print("Oh no! That's not a valid value. Try again...")
except NameError as err:
print("({})".format(err))
else:
print("Each person owes ${}".format(amount_due))