Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial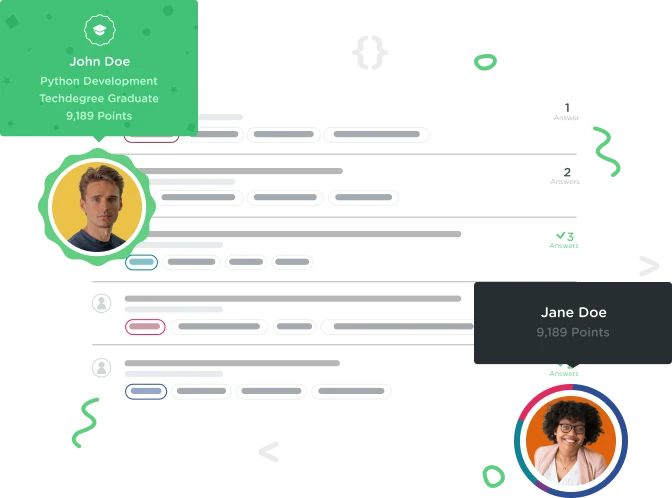

Gabriel Tutia
2,652 PointsOvercomplicated enum
I wrote the enum like this:
enum Icon: String {
case ClearDay = "clear-day"
case ClearNight = "clear-night"
case Rain = "rain"
case Snow = "snow"
case Sleet = "sleet"
case Wind = "wind"
case Fog = "fog"
case Cloudy = "cloudy"
case PartlyCloudyDay = "partly-cloudy-day"
case PartlyCloudyNight = "partly-cloudy-night"
func toImage () -> UIImage?{
let imageName = self.rawValue
return UIImage(named: imageName)
}
}
And it worked! So I was wondering: isn't all this switch part unnecessary and against the DRY principle?
(I'm asking in case there is a reason for switching and I haven't noticed)
3 Answers

Aubrey Taylor
2,991 PointsYou're not the only one to think this: https://teamtreehouse.com/community/why-arent-we-using-the-raw-values-of-the-enum
I think the instructor here is trying to keep things simple while illustrating the usefulness of Enums. In this case, the implementation may have ended up slightly contrived for the tutorials sake. Of course we are all encouraged to investigate alternative approaches.
I too arrived at a similar approach, with the small addition of appending ".png" to the string returned by .rawValue
.
My approach looked like this:
enum Icon: String {
case ClearDay = "clear-day"
case ClearNight = "clear-night"
case Rain = "rain"
case Snow = "snow"
case Sleet = "sleet"
case Wind = "wind"
case Fog = "fog"
case Cloudy = "cloudy"
case PartlyCloudyDay = "partly-cloudy-day"
case PartlyCloudyNight = "partly-cloudy-night"
case Default = "default"
func toImage() -> UIImage? {
return UIImage(named: "\(self.rawValue).png")
}
}
Additionally I switched up the icon init to allow the let
keyword (the instructor mentions this in the video)
if let iconString = weatherDictionary["icon"] as? String,
let weatherIcon: Icon = Icon(rawValue: iconString) {
icon = weatherIcon.toImage()
} else {
icon = Icon(rawValue: "default")?.toImage()
}
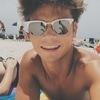
garyguermantokman
21,097 PointsThe switch statement assigns the proper image to the UIImageView depending on the data retrieved from the weather API. Without the switch statement, we would not know which icon would be assigned to the corresponding weather icon from the weather API. If it meets the certain case that image is assigned to the UIImageView.

Aubrey Taylor
2,991 PointsThe raw value of the enums here are conveniently mapped to the actual file names in our .xcassets bundle. You would only need the switch here if that wasn't true. In our case we can streamline the implementation as Gabriel has above or as I have below.
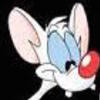
Jake Adams
1,608 PointsIt seems you are mixing values. Usually I start to question myself when I see repetition in an enum. You might consider 2 enums (or more):
enum TimeOfDay {
case Day
case Night
}
enum Weather {
case Rain
case Snow
case Sleet
}
As I look at this, I could see having a Precipitation enum, a Sky enum (clear, cloudy, foggy). With associated values, you could wrangle these in to something pretty flexible. In your current enum, you would have to add values for every possible combination. What happens when it's raining and foggy? Or a cloudy night vs day?
This is a good course on enums: https://teamtreehouse.com/library/swift-20-enumerations-and-optionals
Hope this helps a little!

Aubrey Taylor
2,991 PointsThis answer isn't really related to the question. The user is asking how to create a simplified enum based on the implementation described in the video. Though I agree with you that thoughtful naming is helpful in general.