Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial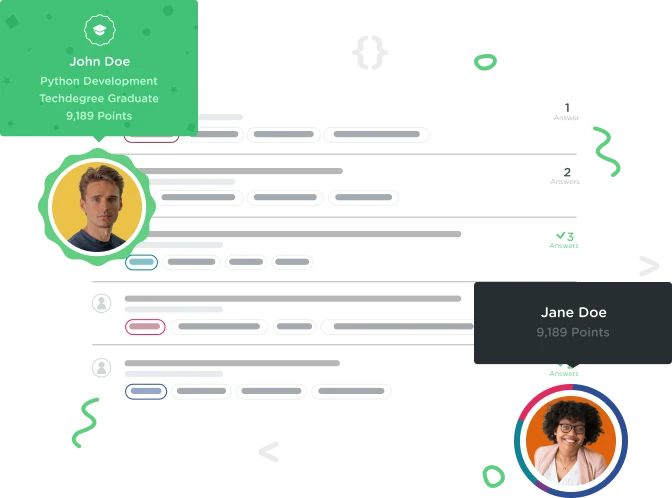

Saul Glasman
18,284 PointsOverflow menu not showing in toolbar
I just completed the golf scorecard assignment from the Android activity lifecycles course, and I tried to set up the app bar and menu in accordance with Google's instructions at https://developer.android.com/training/appbar/setting-up. Although I can get the title and app bar to display, the overflow menu simply isn't showing up. Looking around on the internet, I can see that many people have had similar problems, but none of the simple fixes I see suggested have worked for me.
I'm including my MainActivity java and xml files, as well as the menu xml, below:
package com.saulglasman.golfscorecard;
import android.content.SharedPreferences;
import android.databinding.DataBindingUtil;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.support.v7.widget.DividerItemDecoration;
import android.support.v7.widget.LinearLayoutManager;
import android.support.v7.widget.Toolbar;
import android.view.Menu;
import com.saulglasman.golfscorecard.databinding.ActivityMainBinding;
import java.util.ArrayList;
import java.util.List;
public class MainActivity extends AppCompatActivity {
private static final String PREFS_FILE = "com.saulglasman.golfscorecard.preferences";
private GolfAdapter adapter;
private ActivityMainBinding binding;
private List<Hole> holes = initHoleList();
private Toolbar toolbar;
private SharedPreferences sharedPreferences;
private SharedPreferences.Editor editor;
private List<Hole> initHoleList() {
holes = new ArrayList<>();
for (int i = 1; i <= 18; i++) {
holes.add(new Hole(i));
}
return holes;
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
toolbar = findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
sharedPreferences = getSharedPreferences(PREFS_FILE, MODE_PRIVATE);
editor = sharedPreferences.edit();
for (int i = 0; i < 18; i++) {
holes.get(i).setScore(sharedPreferences.getInt(String.valueOf(i), 0 ));
}
binding = DataBindingUtil.setContentView(this, R.layout.activity_main);
adapter = new GolfAdapter(holes);
binding.holesList.setAdapter(adapter);
binding.holesList.setHasFixedSize(true);
binding.holesList.addItemDecoration(new DividerItemDecoration(this,
DividerItemDecoration.VERTICAL));
binding.holesList.setLayoutManager(new LinearLayoutManager(this));
}
@Override
protected void onPause() {
super.onPause();
for (int i = 0; i < 18; i++) {
editor.putInt(String.valueOf(i), holes.get(i).getScore());
editor.apply();
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.menu, menu);
return true;
}
}
<?xml version="1.0" encoding="utf-8"?>
<layout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools">
<android.support.constraint.ConstraintLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<android.support.v7.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="0dp"
android:layout_height="?attr/actionBarSize"
android:background="?attr/colorPrimary"
android:theme="@style/ThemeOverlay.AppCompat.ActionBar"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:popupTheme="@style/ThemeOverlay.AppCompat.Light"
app:title="Scoreboard"
app:titleTextColor="@android:color/white" />
<android.support.v7.widget.RecyclerView
android:id="@+id/holesList"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_margin="0dp"
android:paddingEnd="16dp"
android:paddingStart="16dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/toolbar"/>
</android.support.constraint.ConstraintLayout>
</layout>
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
tools:context="com.saulglasman.golfscorecard.MainActivity">
<item android:id="@+id/action_settings"
android:title = "Action settings"
app:showAsAction="never"/>
</menu>