Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial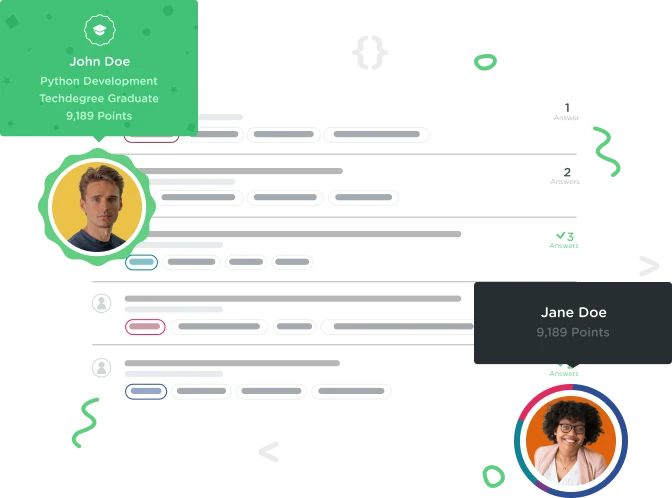

Noah Verkaik
10,952 PointsOverride the Scan method to return true if any value in the array is a repeat of the value immediately preceding it.
This should do it, right??
namespace Treehouse.CodeChallenges
{
class SequenceDetector
{
public virtual bool Scan(int[] sequence)
{
return true;
}
}
}
namespace Treehouse.CodeChallenges
{
class RepeatDetector : SequenceDetector
{
public override bool Scan(int[] sequence)
{
for(int i = 0; i < sequence.Length; i++){
if(sequence[i] == sequence[i+1])
{
return true;
} else {return false;}
}
return true;
}
}
}
2 Answers
Robin Card
9,977 PointsHi,
There's two problems. Firstly, when you check the item that is last in the array, it will try and check if that matches the next item which doesn't exist.
Secondly, your if/then/else statement is returning true or false when it checks the very first item in the array. You want it to default to false, but return true the moment a match is found. So you don't need the else at all.
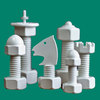
Steven Parker
231,268 PointsI can see two issues:
- you won't want to return false until after the loop (otherwise you only check one pair)
- you will need to restrict the loop so that you don't attempt to access an item beyond the length