Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial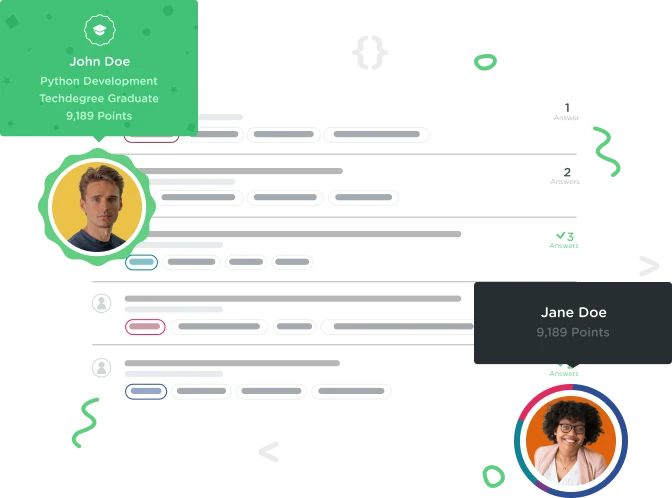

Kamran Ismayilov
5,753 PointsOverriding
import UIKit
class Product { var title: String = "" var price: Double = 0.0
init(title: String, price: Double) {
self.title = title
self.price = price
}
func discountedPrice(percentage: Double) -> Double {
return price - (price * percentage / 100)
}
}
enum Size { case Small, Medium, Large
init() {
self = .Small
}
}
class Clothing: Product {
var size = Size()
override func discountedPrice(percentage: Double = 10.0) -> Double {
return super.discountedPrice(percentage)
}
var tshirt = Clothing(title: "T-shirt", price: 29.99)
tshirt.size tshirt.title tshirt.discountedPrice(10)
let quadcopter = Product(title: "Quadcopter", price: 499)
I did the same as on the video, but got an error pointing to t-shirt.size and also at the and saying "Expected declaration"
also when wanted to add an underscore before percentage in my override function it again complained saying " Extraneous '_' in parameter: 'percentage' has no keyword argument "
Is it because of lately update of Xcode? if yes what is solution? Thanks in advance!
6 Answers
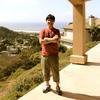
Richard Lu
20,185 PointsNormally, what you mentioned with the underscore would work, but unfortunately with the recent update it might of affected it. My approach to remedy the issue would be to create another method that calls the inherited method.
func getMeDiscountedPrice(percentage: Double = 10.0) -> Double {
return discountedPrice(percentage)
}
This isn't as clean as what Amit had, but it works.
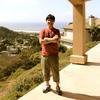
Richard Lu
20,185 PointsThe problem that you're having is this function:
override func discountedPrice(percentage: Double = 10.0) -> Double {
return super.discountedPrice(percentage)
}
The compiler is trying to find a method signature that it can override within it's super class. The signature includes:
- Methods name
- Parameters
- Return type
source: http://tinyurl.com/qaffx34
In your case, the parameters default value is not the same as the one you're trying to override.
I hope I've helped :)
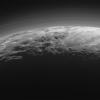
tremaine
2,645 PointsThanks Richard!! That worked for me.

Kamran Ismayilov
5,753 Pointsand what should I do ?? I did the same as was sown on the video. How to correct it?

Kamran Ismayilov
5,753 PointsIt did not work, still says 'Expected declaration'
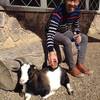
Stamford Hwang
3,329 PointsIt looks like your "class Clothing: Product" is missing a closing }. Try that, and see if it works.